vs2019与sq serverl数据库连接实现增删修改
时间: 2024-05-11 15:18:57 浏览: 143
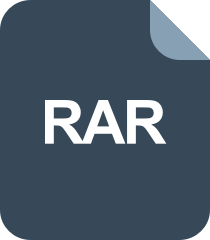
VS实现SQL数据库的增删改查

要在VS2019中与SQL Server数据库进行连接,您需要使用SQL Server Management Studio(SSMS)将数据库创建并设置连接字符串。一旦您完成了这些步骤,您可以使用ADO.NET或Entity Framework等ORM工具来连接和操作数据库。
以下是一个使用ADO.NET进行增删改查操作的示例:
1. 首先,您需要在项目中添加一个连接字符串。在App.config文件中添加以下代码:
```
<connectionStrings>
<add name="MyConnectionString" connectionString="Data Source=<serverName>;Initial Catalog=<databaseName>;User ID=<username>;Password=<password>" providerName="System.Data.SqlClient" />
</connectionStrings>
```
请将serverName、databaseName、username和password替换为您的数据库服务器名称、数据库名称、用户名和密码。
2. 创建一个数据库操作类。例如,以下是一个名为“CustomerDAL”的类,其中包含一些常见的增删改查操作:
```
public class CustomerDAL
{
private string connectionString = ConfigurationManager.ConnectionStrings["MyConnectionString"].ConnectionString;
public List<Customer> GetAllCustomers()
{
List<Customer> customers = new List<Customer>();
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "SELECT * FROM Customers";
SqlCommand command = new SqlCommand(query, connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Customer customer = new Customer();
customer.CustomerID = reader["CustomerID"].ToString();
customer.CompanyName = reader["CompanyName"].ToString();
customer.ContactName = reader["ContactName"].ToString();
customer.ContactTitle = reader["ContactTitle"].ToString();
customer.Address = reader["Address"].ToString();
customer.City = reader["City"].ToString();
customer.Region = reader["Region"].ToString();
customer.PostalCode = reader["PostalCode"].ToString();
customer.Country = reader["Country"].ToString();
customer.Phone = reader["Phone"].ToString();
customer.Fax = reader["Fax"].ToString();
customers.Add(customer);
}
reader.Close();
}
return customers;
}
public void AddCustomer(Customer customer)
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "INSERT INTO Customers (CustomerID, CompanyName, ContactName, ContactTitle, Address, City, Region, PostalCode, Country, Phone, Fax) VALUES (@CustomerID, @CompanyName, @ContactName, @ContactTitle, @Address, @City, @Region, @PostalCode, @Country, @Phone, @Fax)";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@CustomerID", customer.CustomerID);
command.Parameters.AddWithValue("@CompanyName", customer.CompanyName);
command.Parameters.AddWithValue("@ContactName", customer.ContactName);
command.Parameters.AddWithValue("@ContactTitle", customer.ContactTitle);
command.Parameters.AddWithValue("@Address", customer.Address);
command.Parameters.AddWithValue("@City", customer.City);
command.Parameters.AddWithValue("@Region", customer.Region);
command.Parameters.AddWithValue("@PostalCode", customer.PostalCode);
command.Parameters.AddWithValue("@Country", customer.Country);
command.Parameters.AddWithValue("@Phone", customer.Phone);
command.Parameters.AddWithValue("@Fax", customer.Fax);
connection.Open();
command.ExecuteNonQuery();
}
}
public void UpdateCustomer(Customer customer)
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "UPDATE Customers SET CompanyName=@CompanyName, ContactName=@ContactName, ContactTitle=@ContactTitle, Address=@Address, City=@City, Region=@Region, PostalCode=@PostalCode, Country=@Country, Phone=@Phone, Fax=@Fax WHERE CustomerID=@CustomerID";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@CustomerID", customer.CustomerID);
command.Parameters.AddWithValue("@CompanyName", customer.CompanyName);
command.Parameters.AddWithValue("@ContactName", customer.ContactName);
command.Parameters.AddWithValue("@ContactTitle", customer.ContactTitle);
command.Parameters.AddWithValue("@Address", customer.Address);
command.Parameters.AddWithValue("@City", customer.City);
command.Parameters.AddWithValue("@Region", customer.Region);
command.Parameters.AddWithValue("@PostalCode", customer.PostalCode);
command.Parameters.AddWithValue("@Country", customer.Country);
command.Parameters.AddWithValue("@Phone", customer.Phone);
command.Parameters.AddWithValue("@Fax", customer.Fax);
connection.Open();
command.ExecuteNonQuery();
}
}
public void DeleteCustomer(string customerID)
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "DELETE FROM Customers WHERE CustomerID=@CustomerID";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@CustomerID", customerID);
connection.Open();
command.ExecuteNonQuery();
}
}
}
```
3. 在您的应用程序中使用该类进行操作。例如,以下是一个使用该类获取所有客户的示例:
```
CustomerDAL customerDAL = new CustomerDAL();
List<Customer> customers = customerDAL.GetAllCustomers();
```
4. 您还可以使用该类进行其他操作,例如添加、更新和删除客户。例如,以下是向数据库中添加一个新客户的示例:
```
CustomerDAL customerDAL = new CustomerDAL();
Customer customer = new Customer();
customer.CustomerID = "ALFKI";
customer.CompanyName = "Alfreds Futterkiste";
customer.ContactName = "Maria Anders";
customer.ContactTitle = "Sales Representative";
customer.Address = "Obere Str. 57";
customer.City = "Berlin";
customer.Region = null;
customer.PostalCode = "12209";
customer.Country = "Germany";
customer.Phone = "030-0074321";
customer.Fax = "030-0076545";
customerDAL.AddCustomer(customer);
```
请注意,这只是一个示例,并且您需要根据您的数据库模式和需要进行适当的修改和调整。
阅读全文
相关推荐




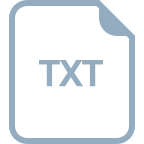
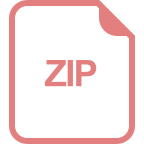
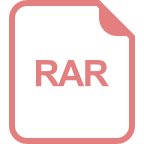
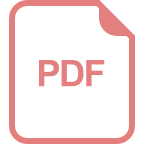
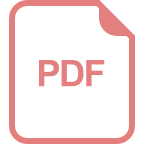
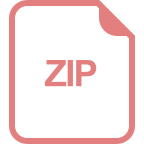
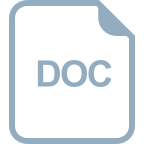