基于Socket通信的电商客服系统设计与实现 基本功能要求: (1)用户端: a)可以发起连接,等待客服端响应,客服端长时间未响应,需要显示客服忙提示; b)除了可以发送文字外,还可以发送图片; (2)客服端: a)可以同时服务多个用户,有新的用户申请服务时有提示,可以选择服务或者拒绝服务; b)除了可以发送文字外,还可以发送文件给用户; 聊天记录需要保存在本地一个文件,同一个用户再次申请聊天时,需要打开文件,导入聊天记录,可以在聊天框查看之前记录;
时间: 2024-04-03 12:31:29 浏览: 97
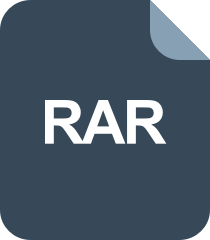
Socket 客服端
以下是基于Socket通信的电商客服系统的Java实现代码:
1. 用户端代码
```java
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import java.net.Socket;
public class UserClient extends JFrame implements ActionListener {
private JTextArea textArea;
private JTextField textField;
private JButton connectButton;
private JButton sendButton;
private JButton imageButton;
private Socket socket;
private DataInputStream dis;
private DataOutputStream dos;
public UserClient() {
setTitle("用户客户端");
setSize(500, 500);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
textArea = new JTextArea();
textArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new BoxLayout(bottomPanel, BoxLayout.X_AXIS));
textField = new JTextField();
bottomPanel.add(textField);
connectButton = new JButton("连接客服");
connectButton.addActionListener(this);
bottomPanel.add(connectButton);
sendButton = new JButton("发送");
sendButton.addActionListener(this);
bottomPanel.add(sendButton);
imageButton = new JButton("发送图片");
imageButton.addActionListener(this);
bottomPanel.add(imageButton);
add(bottomPanel, "South");
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == connectButton) {
try {
socket = new Socket("localhost", 8888);
dis = new DataInputStream(socket.getInputStream());
dos = new DataOutputStream(socket.getOutputStream());
textArea.append("已连接客服\n");
} catch (IOException ex) {
textArea.append("连接失败,请重试\n");
}
} else if (e.getSource() == sendButton) {
String message = textField.getText();
try {
dos.writeUTF(message);
textArea.append("我:" + message + "\n");
textField.setText("");
} catch (IOException ex) {
textArea.append("发送失败,请重试\n");
}
} else if (e.getSource() == imageButton) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
FileInputStream fis = new FileInputStream(file);
byte[] buffer = new byte[(int) file.length()];
fis.read(buffer);
dos.writeInt(buffer.length);
dos.write(buffer);
textArea.append("已发送图片:" + file.getName() + "\n");
} catch (IOException ex) {
textArea.append("发送失败,请重试\n");
}
}
}
}
public void run() {
while (true) {
try {
String message = dis.readUTF();
textArea.append(message + "\n");
} catch (IOException e) {
textArea.append("连接已断开\n");
break;
}
}
}
public static void main(String[] args) {
UserClient userClient = new UserClient();
userClient.setVisible(true);
userClient.run();
}
}
```
2. 客服端代码
```java
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class Server extends JFrame implements ActionListener {
private JTextArea textArea;
private JButton startButton;
private JButton stopButton;
private ServerSocket serverSocket;
private Map<String, Socket> socketMap = new HashMap<>();
private Map<String, DataInputStream> disMap = new HashMap<>();
private Map<String, DataOutputStream> dosMap = new HashMap<>();
public Server() {
setTitle("客服服务器");
setSize(500, 500);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
textArea = new JTextArea();
textArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new BoxLayout(bottomPanel, BoxLayout.X_AXIS));
startButton = new JButton("启动服务器");
startButton.addActionListener(this);
bottomPanel.add(startButton);
stopButton = new JButton("停止服务器");
stopButton.addActionListener(this);
stopButton.setEnabled(false);
bottomPanel.add(stopButton);
add(bottomPanel, "South");
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
try {
serverSocket = new ServerSocket(8888);
textArea.append("服务器已启动\n");
startButton.setEnabled(false);
stopButton.setEnabled(true);
new Thread(() -> {
while (true) {
try {
Socket socket = serverSocket.accept();
DataInputStream dis = new DataInputStream(socket.getInputStream());
String userId = dis.readUTF();
if (socketMap.containsKey(userId)) {
DataOutputStream dos = dosMap.get(userId);
dos.writeUTF("客服忙,请稍后再试");
} else {
socketMap.put(userId, socket);
disMap.put(userId, dis);
DataOutputStream dos = new DataOutputStream(socket.getOutputStream());
dosMap.put(userId, dos);
textArea.append(userId + "已连接\n");
new Thread(() -> {
while (true) {
try {
int length = dis.readInt();
byte[] buffer = new byte[length];
dis.readFully(buffer);
for (Map.Entry<String, DataOutputStream> entry : dosMap.entrySet()) {
if (!entry.getKey().equals(userId)) {
entry.getValue().writeInt(length);
entry.getValue().write(buffer);
}
}
textArea.append(userId + "已发送图片\n");
} catch (IOException ex) {
textArea.append(userId + "连接已断开\n");
socketMap.remove(userId);
disMap.remove(userId);
dosMap.remove(userId);
break;
}
}
}).start();
}
} catch (IOException ex) {
textArea.append("客户端连接失败\n");
}
}
}).start();
} catch (IOException ex) {
textArea.append("服务器启动失败\n");
}
} else if (e.getSource() == stopButton) {
try {
serverSocket.close();
Set<String> userIdSet = socketMap.keySet();
for (String userId : userIdSet) {
Socket socket = socketMap.get(userId);
socket.close();
}
textArea.append("服务器已停止\n");
startButton.setEnabled(true);
stopButton.setEnabled(false);
} catch (IOException ex) {
textArea.append("服务器停止失败\n");
}
}
}
public static void main(String[] args) {
Server server = new Server();
server.setVisible(true);
}
}
```
在运行客户端和服务器端之前,需要先启动服务器端。在启动服务器端之后,可以启动多个客户端,每个客户端可以输入自己的ID号,然后连接到服务器端。客户端可以发送文字消息和图片消息,客服端可以接收到所有客户端发送的消息,并向每个客户端发送文件和文字消息。
以上是基于Socket通信的电商客服系统的Java实现代码,你可以根据需要进行修改和扩展。
阅读全文
相关推荐
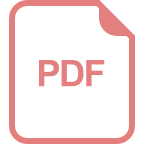


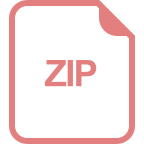
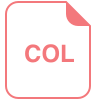
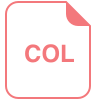
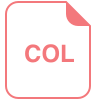
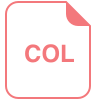
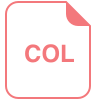
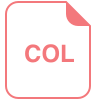
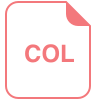
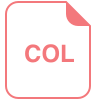
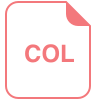
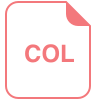
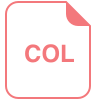
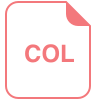
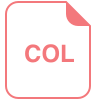