单片机api在线升级软件C语言代码实现
时间: 2024-12-10 12:34:32 浏览: 15
单片机API在线升级通常涉及到通过串口、网络或其他通信方式下载新的程序到硬件中替换现有的固件。由于C语言常用于编写单片机应用程序,这里提供一个简单的示例,展示如何在C语言中处理基本的API升级过程:
```c
#include <stdio.h>
#include <string.h> // 包含字符串操作函数
#include "communication.h" // 假设有一个负责串口通信的通信库
// 原始API结构体
typedef struct {
uint8_t version;
void (*function_ptr)(void);
} OldApi;
// 新API结构体
typedef struct {
uint8_t version;
void (*function_ptr)(void);
char update_url[50]; // 更新URL地址
} NewApi;
// 检查并更新API函数
void check_and_upgrade_api(OldApi *old_api, NewApi *new_api) {
if (old_api->version != new_api->version) {
printf("New API version available, downloading...\n");
// 这里需要连接到服务器下载新API
download_from_url(new_api->update_url, (uint8_t*)old_api); // 假设download_from_url是一个模拟函数
old_api->function_ptr = new_api->function_ptr; // 更新函数指针
printf("Upgrade successful.\n");
} else {
printf("Current API is up to date.\n");
}
}
int main(void) {
OldApi old_api = {0, some_function}; // 假设some_function是原始API的入口点
NewApi new_api = {1, another_function, "http://example.com/new_api.hex"};
check_and_upgrade_api(&old_api, &new_api);
return 0;
}
```
在这个例子中,`check_and_upgrade_api`函数比较旧API版本和新API版本,如果不同,则从服务器下载新API并替换原有的函数指针。请注意,这只是一个简化版的示例,实际的单片机升级过程中还需要考虑错误处理、序列化和反序列化数据、以及可能的固件分区等问题。
阅读全文
相关推荐



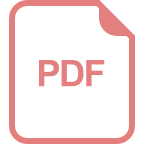














