1. 定义复数(Complex)类 。 要求: (1)复数有实部和虚部属性; (2)定义类的构造方法; (3)实现复数加减法并输出复数属性的值。 (4)重载toString方法,用于对象属性值的输出。
时间: 2024-02-18 21:00:21 浏览: 26
好的,以下是一个简单的复数类的实现:
```C++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double real, double imag) {
this->real = real;
this->imag = imag;
}
// 复数加法
Complex add(Complex other) {
double newReal = this->real + other.real;
double newImag = this->imag + other.imag;
return Complex(newReal, newImag);
}
// 复数减法
Complex subtract(Complex other) {
double newReal = this->real - other.real;
double newImag = this->imag - other.imag;
return Complex(newReal, newImag);
}
// 重载 toString 方法
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << " + " << c.imag << "i";
return os;
}
};
```
这个类有一个构造方法,用于初始化实部和虚部。它还有两个方法,用于实现复数的加减法。最后,它重载了 << 运算符,用于输出对象属性值。你可以通过下面的代码来测试这个类:
```C++
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex sum = c1.add(c2);
Complex diff = c1.subtract(c2);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "sum = " << sum << endl;
cout << "diff = " << diff << endl;
return 0;
}
```
输出结果应该是:
```
c1 = 1 + 2i
c2 = 3 + 4i
sum = 4 + 6i
diff = -2 - 2i
```
相关推荐
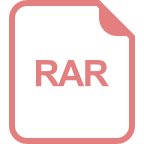
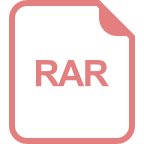
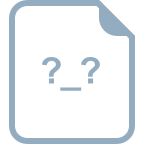










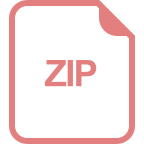
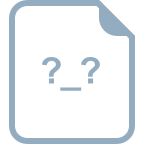