python定义一个复数类、并实现以下复数类的方法_定义一个复数类,并实现以下复数类的方法:构造方法、得到实部、得到虚部、设置实部、设置虚部、复数的加...
时间: 2023-08-06 17:05:24 浏览: 43
好的,我可以为你解答这个问题。以下是Python定义一个复数类,并实现以下复数类方法的示例代码:
```python
class ComplexNumber:
def __init__(self, real_part, imaginary_part):
self.real_part = real_part
self.imaginary_part = imaginary_part
def get_real_part(self):
return self.real_part
def get_imaginary_part(self):
return self.imaginary_part
def set_real_part(self, new_real_part):
self.real_part = new_real_part
def set_imaginary_part(self, new_imaginary_part):
self.imaginary_part = new_imaginary_part
def add(self, other):
new_real_part = self.real_part + other.real_part
new_imaginary_part = self.imaginary_part + other.imaginary_part
return ComplexNumber(new_real_part, new_imaginary_part)
def subtract(self, other):
new_real_part = self.real_part - other.real_part
new_imaginary_part = self.imaginary_part - other.imaginary_part
return ComplexNumber(new_real_part, new_imaginary_part)
def multiply(self, other):
new_real_part = self.real_part * other.real_part - self.imaginary_part * other.imaginary_part
new_imaginary_part = self.real_part * other.imaginary_part + self.imaginary_part * other.real_part
return ComplexNumber(new_real_part, new_imaginary_part)
def divide(self, other):
denominator = float(other.real_part ** 2 + other.imaginary_part ** 2)
new_real_part = (self.real_part * other.real_part + self.imaginary_part * other.imaginary_part) / denominator
new_imaginary_part = (-self.real_part * other.imaginary_part + self.imaginary_part * other.real_part) / denominator
return ComplexNumber(new_real_part, new_imaginary_part)
def __str__(self):
return "{} + {}i".format(self.real_part, self.imaginary_part)
```
在这个示例中,我们定义了一个名为`ComplexNumber`的复数类。构造方法接受实部和虚部作为参数,并将它们存储在对象内部。我们还定义了一些其他方法,例如获取和设置实部和虚部的方法,以及复数的加、减、乘和除法。
我们还重写了`__str__`方法,以便在打印复数对象时输出易于阅读的字符串表示形式。
以下是使用该类的示例演示:
```python
# 创建两个复数对象
c1 = ComplexNumber(2, 3)
c2 = ComplexNumber(4, -5)
# 获取实部和虚部
print(c1.get_real_part()) # 输出 2
print(c1.get_imaginary_part()) # 输出 3
# 设置实部和虚部
c1.set_real_part(5)
c1.set_imaginary_part(-2)
print(c1) # 输出 5 - 2i
# 加、减、乘和除复数
c3 = c1.add(c2)
print(c3) # 输出 9 - 2i
c4 = c1.subtract(c2)
print(c4) # 输出 1 + 7i
c5 = c1.multiply(c2)
print(c5) # 输出 22 - 7i
c6 = c1.divide(c2)
print(c6) # 输出 -0.08 + 0.56i
```
相关推荐
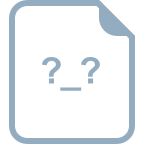
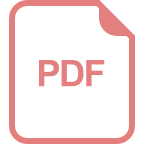














