python定义一个复数类_Python中complex复数类型的简单介绍
时间: 2024-02-19 11:02:31 浏览: 168
复数是由实部和虚部组成的数,可以用数学中的符号“i”表示虚数单位,即i^2 = -1。在Python中,复数可以使用complex类型表示。complex类型是由实数和虚数组成的,实数和虚数部分都是浮点数类型。
Python中complex类型的表示方法为:real + imag * 1j,其中real表示实数部分,imag表示虚数部分,1j表示虚数单位。
例如,complex(2, 3)表示实部为2,虚部为3的复数2+3i。
我们也可以使用加减乘除等数学运算符来进行复数的加减乘除运算,例如:
```python
a = complex(2, 3)
b = complex(4, 5)
print(a + b) # 输出(6+8j)
print(a - b) # 输出(-2-2j)
print(a * b) # 输出(-7+22j)
print(a / b) # 输出(0.5609756097560976+0.0487804878048781j)
```
Python中还提供了一些内置函数用于处理复数,例如abs()函数可以计算复数的模(即大小),例如:
```python
a = complex(2, 3)
print(abs(a)) # 输出3.605551275463989
```
除此之外,Python中还有一些标准库,例如cmath库,提供了更多的复数运算函数,例如对数、幂、三角函数等等。
相关问题
python定义一个复数类complex
Python中已经有一个内置的复数类complex,可以直接使用。如果需要自定义一个复数类,可以按照以下方式定义:
```python
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __add__(self, other):
return Complex(self.real + other.real, self.imag + other.imag)
def __sub__(self, other):
return Complex(self.real - other.real, self.imag - other.imag)
def __mul__(self, other):
return Complex(self.real * other.real - self.imag * other.imag, self.real * other.imag + self.imag * other.real)
def __truediv__(self, other):
denominator = other.real ** 2 + other.imag ** 2
return Complex((self.real * other.real + self.imag * other.imag) / denominator, (self.imag * other.real - self.real * other.imag) / denominator)
def __str__(self):
return f"{self.real} + {self.imag}i"
```
这个类包含了四个基本的运算方法:加、减、乘、除。其中加、减、乘的实现比较简单,除法需要用到复数的共轭。同时,为了方便输出,还定义了一个__str__方法。
python定义一个复数类complex、并实现复数相加
### 回答1:
Python中定义了一个复数类complex,可以通过使用该类创建复数对象并进行复数相加运算。例如以下代码:
```python
c1 = complex(3, 4) # 创建一个复数3+4j
c2 = complex(1, 2) # 创建一个复数1+2j
c3 = c1 + c2 # 复数相加
print(c3) # 输出结果为(4+6j)
```
其中,`complex`类的参数分别为实部和虚部。可以通过实部和虚部属性(`real`和`imag`)访问复数的实部和虚部。
### 回答2:
Python是一种高级编程语言,它提供了丰富的库和工具,方便程序员快速地进行开发和实现。在Python中,我们可以使用类来定义自己的数据类型,其中包括复数类。
复数是由一个实数和一个虚数构成的数,例如2 + 3i,其中2是实数部分,3i是虚数部分,i是虚数单位。在Python中,可以使用内置的complex函数来创建复数对象,如下所示:
```python
z = complex(2, 3)
print(z) # 输出:(2+3j)
```
创建一个复数类complex,需要定义实数和虚数两个成员变量,并提供构造函数来初始化这两个成员变量,如下所示:
```python
class Complex:
def __init__(self, real=0, imag=0):
self.real = real
self.imag = imag
```
在上面的代码中,我们使用了构造函数__init__来初始化实数和虚数。real和imag是实数和虚数的成员变量,它们的初始值都为0。
然后,我们需要实现复数相加的功能。在复数相加时,需要将两个复数的实数部分和虚数部分分别相加,如下所示:
```python
class Complex:
def __init__(self, real=0, imag=0):
self.real = real
self.imag = imag
def __add__(self, other):
real = self.real + other.real
imag = self.imag + other.imag
return Complex(real, imag)
```
在上面的代码中,我们定义了一个__add__方法来实现复数相加。它接受其他一个复数对象other作为参数,将两个复数的实数和虚数相加,并返回一个新的复数对象。
现在,我们可以创建两个复数对象,然后使用加号操作符进行相加,如下所示:
```python
z1 = Complex(2, 3)
z2 = Complex(4, 5)
z3 = z1 + z2
print(z3.real, z3.imag) # 输出:6 8
```
在上面的代码中,我们使用加号操作符进行复数相加,然后输出结果。
综上所述,我们可以使用Python定义一个复数类complex,然后实现复数相加的功能。在实现过程中,需要定义实数和虚数的成员变量,提供构造函数和__add__方法来初始化成员变量和实现复数相加。
### 回答3:
复数是数学中的一种数形结合物,它由实部和虚部组成。在计算机编程中,我们可以定义一个复数类来表示复数,并实现复数相加。
Python 中已经内置了复数类型,我们可以通过 complex(real, imag) 函数创建一个复数对象。其中 real 表示实部,imag 表示虚部。例如:
```python
z = complex(2, 3)
print(z) # 输出 (2 + 3j)
```
如果我们要自定义一个复数类,可以用 class 关键字定义:
```python
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __add__(self, other):
return Complex(self.real + other.real, self.imag + other.imag)
def __repr__(self):
return '(%s + %sj)' % (self.real, self.imag)
```
在这个类中,我们通过 __init__ 方法定义了实部和虚部,并通过 __add__ 方法实现了两个复数相加。__repr__ 方法用于返回对象的字符串表示。
我们可以使用这个类创建两个复数对象并相加:
```python
a = Complex(2, 3)
b = Complex(4, 5)
c = a + b
print(c) # 输出 (6 + 8j)
```
这样,我们就成功定义了一个复数类并实现了复数相加的操作。在实际编程中,我们可以将复数类和复数运算应用于各种计算场景,具有很强的实用性。
阅读全文
相关推荐
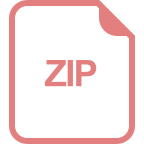
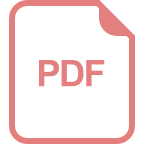
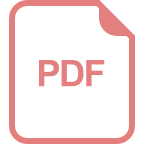












