oauth2.0客户端
时间: 2023-10-02 20:07:10 浏览: 151
OAuth 2.0是一种授权框架,用于授权第三方应用程序访问受保护的资源,而无需共享用户凭据。OAuth 2.0客户端是一个代表应用程序与授权服务器进行交互的实体。
客户端在OAuth 2.0流程中扮演重要角色,它通过向授权服务器发起请求来获取访问令牌(access token)。访问令牌用于向资源服务器请求访问受限资源。客户端通常会将访问令牌存储在安全的位置,以便在需要时使用。
OAuth 2.0客户端通常需要提供以下信息:
1. 客户端ID(Client ID):唯一标识客户端的字符串,由授权服务器分配。
2. 客户端密钥(Client Secret):用于与授权服务器进行身份验证和安全通信的机密字符串。
3. 授权类型(Authorization Grant Type):指定授权流程的类型,常见的类型有授权码模式(Authorization Code Grant)、密码模式(Password Grant)、客户端凭证模式(Client Credentials Grant)等。
根据不同的应用场景和需求,OAuth 2.0客户端可以是Web应用程序、移动应用程序或后端服务等。它们通过与授权服务器进行交互,获取合法的访问令牌,从而访问受保护的资源。
相关问题
oauth2.0客户端获取token
OAuth 2.0是一种授权框架,用于在客户端和资源所有者之间安全地共享资源。在OAuth 2.0中,客户端可以通过授权服务器获取访问令牌(access token),然后使用该访问令牌访问受保护的资源。
以下是客户端获取访问令牌的基本流程:
1. 客户端向授权服务器发送请求,包含客户端标识符和客户端密钥。
2. 授权服务器验证客户端标识符和客户端密钥,如果验证通过,则向客户端返回访问令牌。
3. 客户端使用访问令牌访问受保护的资源。
具体实现方式可以参考OAuth 2.0协议规范,常见的实现方式包括OAuth 2.0的授权码模式、密码模式、客户端模式等。不同的实现方式具体的细节和流程会有所不同,需要根据具体情况进行调整。
java实现oauth2.0 客户端
在 Java 中实现 OAuth2 客户端通常需要使用 OAuth2 客户端库,以下是使用 Spring Security OAuth2 客户端的示例代码:
1. 添加依赖
在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2-client</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
```
2. 配置授权服务器信息
在 application.yml 或 application.properties 文件中添加授权服务器信息:
```yaml
spring:
security:
oauth2:
client:
registration:
my-client:
client-id: my-client-id
client-secret: my-client-secret
authorization-grant-type: authorization_code
redirect-uri: '{baseUrl}/login/oauth2/code/{registrationId}'
scope: user
client-name: My Client
provider:
my-provider:
authorization-uri: https://example.com/oauth2/authorize
token-uri: https://example.com/oauth2/token
user-info-uri: https://example.com/oauth2/userinfo
user-name-attribute: sub
```
其中,`my-client` 和 `my-provider` 是自定义的标识符,用于区分不同的客户端和提供商。`client-id` 和 `client-secret` 是从授权服务器获取的客户端 ID 和密钥。`authorization-grant-type` 指定授权类型,这里是授权码模式。`redirect-uri` 是回调 URL,用于接收授权码。`scope` 是请求的权限范围,这里是请求用户信息。`authorization-uri` 是授权服务器的授权页面 URL,`token-uri` 是获取访问令牌的 URL,`user-info-uri` 是获取用户信息的 URL,`user-name-attribute` 是用户标识符在用户信息中的属性名。
3. 创建登录页面
在 Thymeleaf 模板中创建登录页面:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Login Page</title>
</head>
<body>
<div th:if="${param.error}">
Invalid username and password.
</div>
<div th:if="${param.logout}">
You have been logged out.
</div>
<h2>Login with OAuth2</h2>
<a th:href="@{/oauth2/authorization/my-provider}">Login with My Provider</a>
</body>
</html>
```
其中,`/oauth2/authorization/my-provider` 是登录链接,`my-provider` 是之前配置的提供商标识符。
4. 创建回调页面
在 Thymeleaf 模板中创建回调页面:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>OAuth2 Callback Page</title>
</head>
<body>
<script>
function closeWindow() {
window.opener.location.reload();
window.close();
}
closeWindow();
</script>
</body>
</html>
```
其中,使用 JavaScript 关闭弹出窗口并刷新父窗口。
5. 创建安全配置
创建一个继承 `WebSecurityConfigurerAdapter` 的安全配置类:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/login**", "/webjars/**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.oauth2Login()
.loginPage("/")
.defaultSuccessURL("/home")
.failureUrl("/?error=true")
.and()
.logout()
.logoutSuccessUrl("/")
.permitAll();
}
}
```
其中,`configure()` 方法配置了请求授权规则和 OAuth2 登录的相关参数,`.antMatchers()` 指定了不需要认证的 URL,`.oauth2Login()` 配置了 OAuth2 登录的参数,`.loginPage()` 指定了登录页面 URL,`.defaultSuccessURL()` 指定了登录成功后的默认 URL,`.failureUrl()` 指定了登录失败后的 URL,`.logout()` 配置了注销登录的参数。
6. 创建控制器
创建一个控制器处理回调请求和获取用户信息:
```java
@Controller
public class OAuth2Controller {
@GetMapping("/home")
public String home(Model model, OAuth2AuthenticationToken authentication) {
OAuth2AuthorizedClient client = authorizedClientService.loadAuthorizedClient(authentication.getAuthorizedClientRegistrationId(), authentication.getName());
model.addAttribute("userName", client.getPrincipalName());
return "home";
}
@GetMapping("/login")
public String login() {
return "login";
}
@GetMapping("/logout")
public String logout() {
return "logout";
}
@Autowired
private OAuth2AuthorizedClientService authorizedClientService;
@GetMapping("/login/oauth2/code/{registrationId}")
public String callback(@PathVariable String registrationId, OAuth2AuthenticationToken authentication) {
OAuth2AuthorizedClient client = authorizedClientService.loadAuthorizedClient(registrationId, authentication.getName());
return "redirect:/home";
}
}
```
其中,`home()` 方法获取用户信息并返回到 home.html 模板,`login()` 方法返回到登录页面,`logout()` 方法返回到注销页面,`callback()` 方法处理回调请求并获取 OAuth2 客户端。
这就是使用 Spring Security OAuth2 客户端实现 OAuth2 客户端的基本流程。
阅读全文
相关推荐
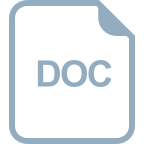
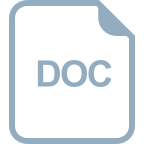
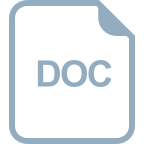
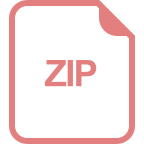
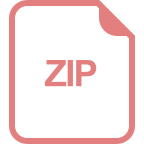
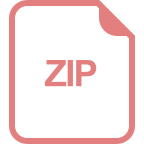
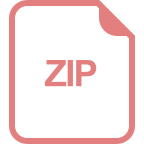
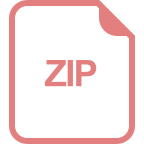
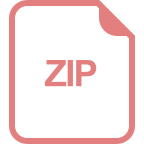
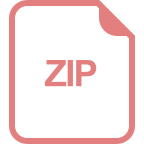
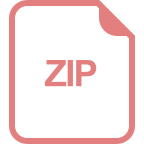
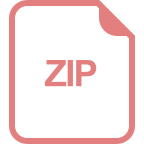
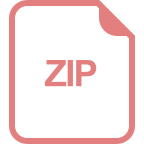
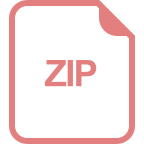
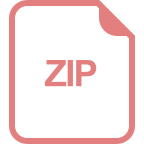
