回溯法求解图着色问题python
时间: 2023-10-14 14:18:27 浏览: 120
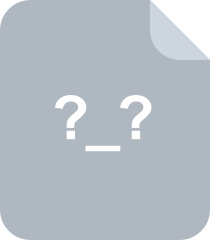
回溯法解决图着色问题

图着色问题是一个经典的组合优化问题,其目标是为给定的无向图中的每个节点分配一种颜色,使得相邻节点之间的颜色不同,并且使用的颜色数最少。
回溯法是解决图着色问题的一种常用方法。具体来说,回溯法通过尝试每一种可能的颜色分配方案来逐步构建解,当发现当前方案不行时,回溯到上一步并尝试其他可能的方案,直到找到满足条件的最优解或者所有的可能性都被尝试过。
以下是一个简单的 Python 代码实现:
```python
def can_color(node, color, graph, colors):
for i in range(len(graph)):
if graph[node][i] == 1 and colors[i] == color:
return False
return True
def graph_coloring(graph, colors, node=0):
if node == len(graph):
return True
for color in colors:
if can_color(node, color, graph, colors):
graph[node][node] = color
if graph_coloring(graph, colors, node+1):
return True
graph[node][node] = 0
return False
if __name__ == '__main__':
graph = [[0, 1, 1, 1],
[1, 0, 1, 0],
[1, 1, 0, 1],
[1, 0, 1, 0]]
colors = [1, 2, 3]
if graph_coloring(graph, colors):
print("Graph can be colored with", len(set([graph[i][i] for i in range(len(graph))])), "colors")
print("Coloring:", [graph[i][i] for i in range(len(graph))])
else:
print("Graph cannot be colored with", len(colors), "colors")
```
该代码中,`graph` 是一个邻接矩阵,表示图的连接关系;`colors` 是可用的颜色列表;`node` 是当前处理的节点。首先,判断当前节点是否已经遍历完所有节点,如果是,则返回 True,表示找到了符合条件的最优解。否则,遍历可用的颜色列表,对每种颜色尝试分配给当前节点,并判断分配后是否满足条件。如果满足条件,则递归处理下一个节点;否则,回溯到上一步并尝试其他可能的颜色分配方案。最终,如果找到了符合条件的最优解,则返回 True,否则返回 False,表示无法找到符合条件的颜色分配方案。
阅读全文
相关推荐


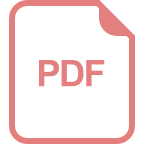
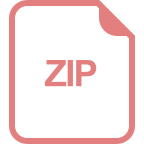
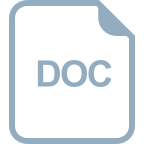
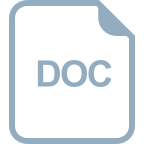
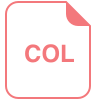
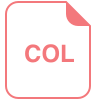
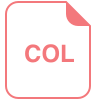
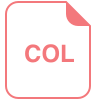
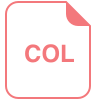
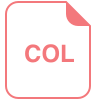
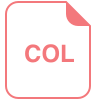
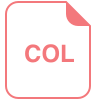
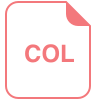