数据结构c语言实现1)根据输入的队列长度n和各元素值建立一个带头结点的循环链表表示的队列(循环链队列),并且只设一个尾指针来指向尾结点,然后输出队列中各元素值。 (2)将数据元素e入队,并输出入队后的队列中各元素值。 (3)将循环链队列的队首元素出队,并输出出队元素的值和出队后队列中各元素值。
时间: 2023-12-14 19:36:43 浏览: 83
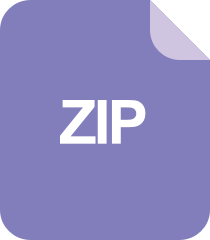
数据结构演示系统 数据结构 C语言实现
1)根据输入的队列长度n和各元素值建立一个带头结点的循环链表表示的队列(循环链队列),并且只设一个尾指针来指向尾结点,然后输出队列中各元素值。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node{
int data;
struct node *next;
}Node, *LinkList;
LinkList CreateList(int n);
void PrintList(LinkList L);
void EnQueue(LinkList L, int e);
int DeQueue(LinkList L);
int main()
{
int n;
printf("Please input the length of the queue: ");
scanf("%d", &n);
LinkList L = CreateList(n);
printf("The queue is: ");
PrintList(L);
return 0;
}
LinkList CreateList(int n)
{
LinkList L = (LinkList)malloc(sizeof(Node));
L->next = L; // 循环链表的头结点指向自己,表示空表
LinkList rear = L; // 尾指针初始化为头结点
for(int i=0; i<n; i++)
{
int data;
printf("Please input the element %d: ", i+1);
scanf("%d", &data);
LinkList p = (LinkList)malloc(sizeof(Node));
p->data = data;
rear->next = p;
rear = p;
}
rear->next = L; // 尾结点指向头结点,形成循环链表
return L;
}
void PrintList(LinkList L)
{
LinkList p = L->next;
while(p != L)
{
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
2)将数据元素e入队,并输出入队后的队列中各元素值。
```c
void EnQueue(LinkList L, int e)
{
LinkList rear = L;
while(rear->next != L) // 找到尾结点
{
rear = rear->next;
}
LinkList p = (LinkList)malloc(sizeof(Node));
p->data = e;
rear->next = p;
p->next = L; // 新插入的结点成为新的尾结点,指向头结点,形成循环链表
}
int main()
{
int n;
printf("Please input the length of the queue: ");
scanf("%d", &n);
LinkList L = CreateList(n);
printf("The queue is: ");
PrintList(L);
int e;
printf("Please input the element to be enqueued: ");
scanf("%d", &e);
EnQueue(L, e);
printf("The queue after enqueue is: ");
PrintList(L);
return 0;
}
```
3)将循环链队列的队首元素出队,并输出出队元素的值和出队后队列中各元素值。
```c
int DeQueue(LinkList L)
{
if(L->next == L) // 空队列
{
printf("The queue is empty.\n");
return -1;
}
LinkList p = L->next;
int e = p->data;
L->next = p->next; // 头结点指向下一个结点,即出队的结点
free(p);
return e;
}
int main()
{
int n;
printf("Please input the length of the queue: ");
scanf("%d", &n);
LinkList L = CreateList(n);
printf("The queue is: ");
PrintList(L);
int e;
printf("Please input the element to be enqueued: ");
scanf("%d", &e);
EnQueue(L, e);
printf("The queue after enqueue is: ");
PrintList(L);
int de_e = DeQueue(L);
printf("The element dequeued is: %d\n", de_e);
printf("The queue after dequeue is: ");
PrintList(L);
return 0;
}
```
阅读全文
相关推荐
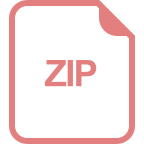















