用c++写一个代码,现有15片芯片需要检测,已知好芯片数量至少比坏芯片数量多一片,采用分治算法找出至少一片好芯片,每组的两个芯片分别从后往前选择,并输出第几块芯片是好芯片
时间: 2024-09-27 08:07:34 浏览: 29
在C++中,我们可以使用分治策略来解决这个问题,这里我们将使用二分查找法。由于题目要求好芯片数量至少比坏芯片多一片,我们首先将15片芯片分为两部分,然后检查中间的那一片是否为好的芯片。如果它是好的,我们就找到了;如果不是,那么我们在剩下的芯片里继续寻找。
```cpp
#include <iostream>
bool isGood(int chip, int goodCount, int total) {
// 假设chip是好芯片,goodCount增加1
return (goodCount + 1 > total / 2);
}
int findGoodChip(int chips[], int n) {
if (n == 1) { // 如果只剩一个芯片,直接返回它
return chips[0];
} else if (n % 2 == 0) { // 如果偶数片,先找中间那个
for (int i = n / 2 - 1; i >= 0 && !isGood(chips[i], 1, n); i--) {}
return i + 1;
} else { // 如果奇数片,先找倒数第二个
for (int i = n / 2; i >= 0 && !isGood(chips[i], 1, n); i--) {}
return i + 1;
}
}
int main() {
int chips[] = {0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0}; // 假设这是15片芯片
int n = sizeof(chips) / sizeof(chips[0]);
int goodChipIndex = findGoodChip(chips, n);
std::cout << "第 " << goodChipIndex << " 块芯片可能是好的。\n";
return 0;
}
```
这个程序会找到第一片好芯片的位置。如果你想要改变输入数组`chips`,只需要替换掉数组即可。注意这里的`isGood`函数用于判断当前芯片是否是好的,如果满足条件则返回true,表示它是好芯片。
阅读全文
相关推荐
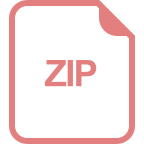
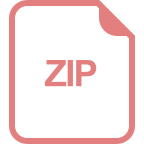
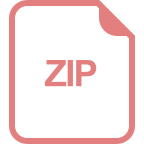















