用C写采用函数统计学生成绩:输入学生的成绩计算并输出这些学生的最低分,最高分和平均分
时间: 2024-09-16 07:05:06 浏览: 41
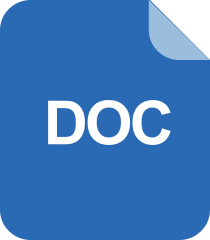
C语言输入学生成绩,计算并输出这些学生的最低分、最高分、平均分。
在C语言中,你可以编写一个函数来完成这个任务。首先,需要一个函数来接收学生成绩的数组,并返回最低分、最高分和平均分。下面是一个基本的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义函数,传入一个整数数组和数组长度
void calculate_scores(int* scores, int n) {
if (n <= 0) {
printf("错误:输入的分数数组为空或长度为0.\n");
return;
}
// 初始化最低分和最高分为数组的第一个元素
int min_score = scores[0];
int max_score = scores[0];
// 遍历数组寻找最小值和最大值
for (int i = 1; i < n; i++) {
if (scores[i] < min_score) {
min_score = scores[i];
}
if (scores[i] > max_score) {
max_score = scores[i];
}
}
// 计算平均分
float sum = 0;
for (int i = 0; i < n; i++) {
sum += scores[i];
}
float average = sum / (float)n;
// 输出结果
printf("最低分: %d\n", min_score);
printf("最高分: %d\n", max_score);
printf("平均分: %.2f\n", average);
}
int main() {
int num_students;
printf("请输入学生人数:");
scanf("%d", &num_students);
// 假设我们有一个动态分配的空间存放学生成绩
int* student_scores = malloc(num_students * sizeof(int));
if (student_scores == NULL) {
printf("内存分配失败.\n");
return 1;
}
// 获取学生成绩并传递给函数
printf("请输入学生成绩:\n");
for (int i = 0; i < num_students; i++) {
scanf("%d", &student_scores[i]);
}
calculate_scores(student_scores, num_students); // 调用函数计算结果
free(student_scores); // 使用完后记得释放内存
return 0;
}
```
在这个程序中,用户会被提示输入学生人数和他们的成绩,然后函数`calculate_scores`会计算并显示最低分、最高分和平均分。
阅读全文
相关推荐
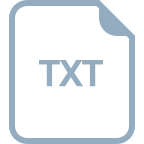
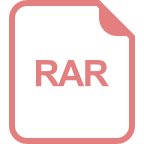















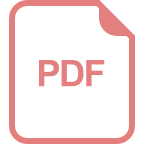