采用函数统计学生成绩:输入多个学生的成绩,计算并输出这些学生的最低分、最高分、平均分。
时间: 2024-09-16 19:05:42 浏览: 72
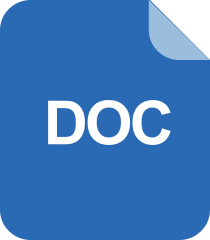
C语言输入学生成绩,计算并输出这些学生的最低分、最高分、平均分。
要编写一个程序来统计多个学生的成绩,你可以创建一个函数来接收学生的成绩数组,并使用其他函数来计算最低分、最高分和平均分。以下是一个简单的C语言实现示例:
```c
#include <stdio.h>
// 函数声明
double find_min(double scores[], int n);
double find_max(double scores[], int n);
double calculate_average(double scores[], int n);
// 主函数
int main() {
int num_students;
double scores[100]; // 假设最多有100个学生
printf("请输入学生数量(不超过100):");
scanf("%d", &num_students);
if (num_students <= 0 || num_students > 100) {
printf("学生数量错误,请重新输入。\n");
return 1;
}
printf("请输入每个学生的分数,用空格隔开:\n");
for (int i = 0; i < num_students; i++) {
scanf("%lf", &scores[i]);
}
double min_score = find_min(scores, num_students);
double max_score = find_max(scores, num_students);
double avg_score = calculate_average(scores, num_students);
printf("最低分:%.2f\n", min_score);
printf("最高分:%.2f\n", max_score);
printf("平均分:%.2f\n", avg_score);
return 0;
}
// 计算最小值的函数
double find_min(double scores[], int n) {
double min = scores[0];
for (int i = 1; i < n; i++) {
if (scores[i] < min) {
min = scores[i];
}
}
return min;
}
// 计算最大值的函数
double find_max(double scores[], int n) {
double max = scores[0];
for (int i = 1; i < n; i++) {
if (scores[i] > max) {
max = scores[i];
}
}
return max;
}
// 计算平均值的函数
double calculate_average(double scores[], int n) {
double sum = 0;
for (int i = 0; i < n; i++) {
sum += scores[i];
}
return sum / n;
}
```
在这个例子中,`find_min()`、`find_max()` 和 `calculate_average()` 分别用于找出给定成绩数组中的最小值、最大值和平均值。`main()` 函数负责获取用户输入并调用这些辅助函数。
如果你想要运行此程序,请复制这段代码到一个名为`stats.c`的文件中,然后使用`gcc stats.c -o stats`编译它,然后执行`./stats`命令。确保你的编译器支持C99标准或更高版本,因为这里使用了`double`类型和动态数组。
阅读全文
相关推荐
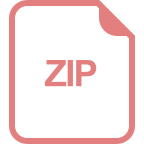
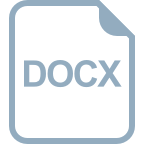














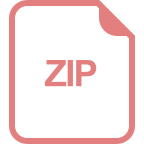
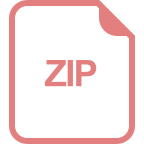
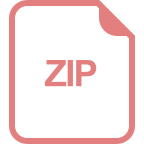