定义学生类,包括学号、姓名、性别、成绩,并将课程成绩全部初始化为0; 定义类成员函数,完成修改学生的信息,统计所有学生课程的平均成绩、最高分和最低分; 输出所有学生信息; 通过输入学生学号或姓名查询某学生的信息。 利用头文件和多个源文件分别实现类的声明、类成员函数的定义和主函数;(20分) 从键盘输入学生数,学生数在2-6之间,如果输入错误,则重新输入。(5分) 定义构造函数完成类成员变量的初始化;由键盘修改学生信息;(20分) 利用静态成员变量与静态成员函数统计所有学生课程的平均成绩、最高分和最低分;(20分) 利用函数的重载实现通过输入学生学号或姓名查询某学生的信息。(20分) 定义析构函数,输出“本程序由+学生姓名+编写!”,如“本程序由张三编写”。(5分)
时间: 2023-05-26 11:07:28 浏览: 162
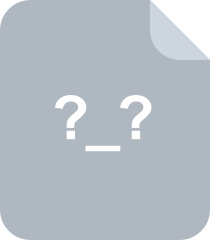
c++定义一个学生类(属性有学号,姓名,成绩),要求输入学生的学号,姓名及三科的成绩,以输入内容来设置学生对象的属性。最后输出学生的学号,姓名及三科的平均成绩。
Student.h
#include <string>
using namespace std;
class Student {
private:
string id;
string name;
char gender;
int score1, score2, score3, score4;
static int count;
static int totalScore;
static int highestScore;
static int lowestScore;
public:
Student(string id, string name, char gender);
~Student();
void updateInfo();
void printInfo();
void searchBy(string keyword);
static void calcAvgScore();
static void calcHighAndLowScore();
};
Student.cpp
#include <iostream>
#include "Student.h"
using namespace std;
int Student::count = 0;
int Student::totalScore = 0;
int Student::highestScore = 0;
int Student::lowestScore = 0;
Student::Student(string id, string name, char gender) {
this->id = id;
this->name = name;
this->gender = gender;
score1 = score2 = score3 = score4 = 0;
count++;
}
Student::~Student() {
cout << "本程序由" << name << "编写!" << endl;
}
void Student::updateInfo() {
cout << "请输入 " << name << " 的成绩:" << endl;
cout << "课程1成绩:";
cin >> score1;
cout << "课程2成绩:";
cin >> score2;
cout << "课程3成绩:";
cin >> score3;
cout << "课程4成绩:";
cin >> score4;
totalScore += score1 + score2 + score3 + score4;
highestScore = max(highestScore, max(score1, max(score2, max(score3, score4))));
lowestScore = min(lowestScore, min(score1, min(score2, min(score3, score4))));
}
void Student::printInfo() {
cout << "学号:" << id << " 姓名:" << name << " 性别:" << gender << " 成绩:"
<< score1 << " " << score2 << " " << score3 << " " << score4 << endl;
}
void Student::searchBy(string keyword) {
if (id == keyword || name == keyword) {
printInfo();
}
}
void Student::calcAvgScore() {
cout << "所有学生的课程平均成绩为:" << (double)totalScore / (count * 4) << endl;
}
void Student::calcHighAndLowScore() {
cout << "所有学生的最高分为:" << highestScore << endl;
cout << "所有学生的最低分为:" << lowestScore << endl;
}
main.cpp
#include <iostream>
#include "Student.h"
using namespace std;
int main() {
int n;
do {
cout << "请输入学生人数(2-6):";
cin >> n;
} while (n < 2 || n > 6);
Student* students[n];
string id, name;
char gender;
for (int i = 0; i < n; i++) {
cout << "请输入第" << i + 1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> id;
cout << "姓名:";
cin >> name;
cout << "性别:";
cin >> gender;
students[i] = new Student(id, name, gender);
}
for (int i = 0; i < n; i++) {
students[i]->updateInfo();
}
Student::calcAvgScore();
Student::calcHighAndLowScore();
string keyword;
cout << "请输入要查询的学生的学号或姓名:";
cin >> keyword;
for (int i = 0; i < n; i++) {
students[i]->searchBy(keyword);
}
for (int i = 0; i < n; i++) {
students[i]->printInfo();
}
for (int i = 0; i < n; i++) {
delete students[i];
}
return 0;
}
阅读全文