Python enumerate函数在教育中的实战:遍历学生数据,轻松实现成绩分析
发布时间: 2024-06-24 18:40:44 阅读量: 5 订阅数: 12 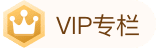
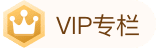
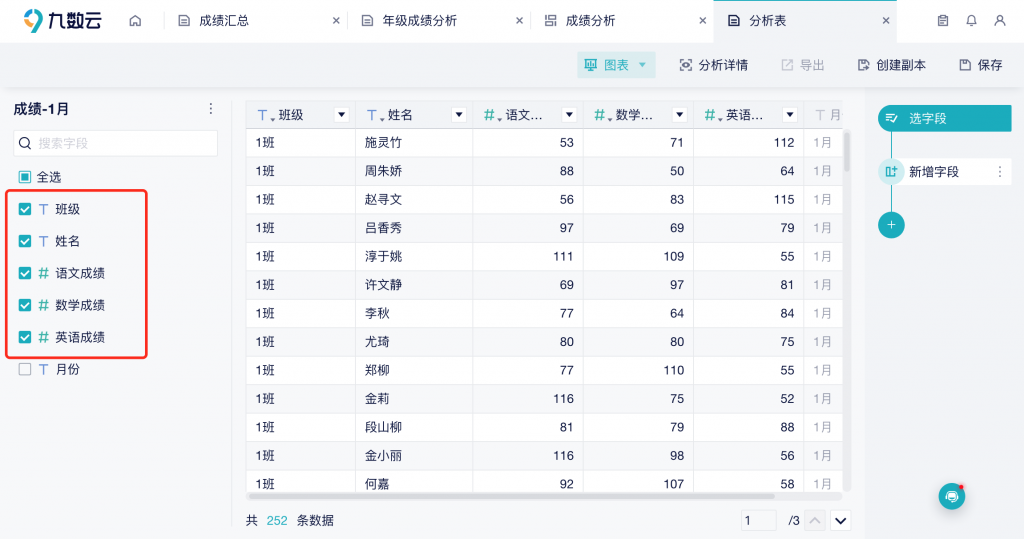
# 1. Python enumerate函数简介和基础用法
Python `enumerate()` 函数是一种内置函数,用于遍历序列(例如列表、元组或字符串),同时返回每个元素的索引和元素本身。其语法如下:
```python
enumerate(sequence, start=0)
```
其中:
* `sequence`:要遍历的序列。
* `start`(可选):遍历的起始索引,默认为 0。
`enumerate()` 函数返回一个枚举对象,该对象是一个迭代器,它包含一个元组,其中第一个元素是索引,第二个元素是序列中的元素。
# 2. enumerate函数在教育中的实战应用
### 2.1 遍历学生数据,实现成绩统计
#### 2.1.1 导入数据并创建学生列表
首先,导入必要的库并加载学生数据:
```python
import pandas as pd
# 加载学生数据
students = pd.read_csv('students.csv')
```
#### 2.1.2 使用enumerate函数遍历学生列表
使用`enumerate()`函数遍历`students`列表,同时获取学生的索引和数据:
```python
# 遍历学生列表
for index, student in enumerate(students.itertuples()):
print(f"Student {index + 1}: {student.name}, Score: {student.score}")
```
#### 2.1.3 统计学生成绩
在遍历过程中,统计每个学生的成绩并存储在`total_score`变量中:
```python
# 统计学生成绩
total_score = 0
for index, student in enumerate(students.itertuples()):
total_score += student.score
```
最终,输出学生的总成绩:
```python
print(f"Total Score: {total_score}")
```
### 2.2 遍历学生数据,实现成绩排序
#### 2.2.1 使用enumerate函数遍历学生列表
使用`enumerate()`函数遍历`students`列表,同时获取学生的索引和数据:
```python
# 遍历学生列表
for index, student in enumerate(students.itertuples()):
print(f"Student {index + 1}: {student.name}, Score: {student.score}")
```
#### 2.2.2 根据成绩对学生进行排序
使用`sorted()`函数根据学生的成绩对列表进行排序,并使用`lambda`函数提取学生的成绩:
```python
# 根据成绩对学生进行排序
sorted_students = sorted(students.itertuples(), key=lambda student: student.score, reverse=True)
```
最终,输出排序后的学生列表:
```python
# 输出排序后的学生列表
for index, student in enumerate(sorted_students):
print(f"Student {index + 1}: {student.name}, Score: {student.score}")
```
# 3.1 遍历字典,同时获取键和值
#### 3.1.1 创建学生字典
```python
# 创建一个学生字典,键为学号,值为学生信息
students = {
'1001': {'name': '小明', 'age': 18, 'score': 90},
'1002': {'name': '小红', 'age': 19, 'score': 85},
'1003': {'name': '小刚', 'age': 20, 'score': 95},
}
```
#### 3.1.2 使用enumerate函数遍历学生字典
```python
# 使用enu
```
0
0
相关推荐
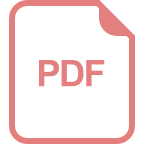
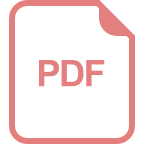
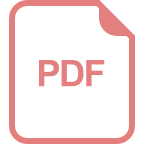
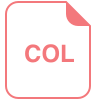
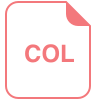
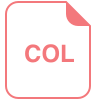
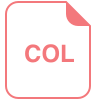

