Python enumerate函数在自然语言处理中的应用:遍历文本数据,提升文本处理效率
发布时间: 2024-06-24 18:25:24 阅读量: 6 订阅数: 16 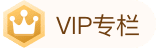
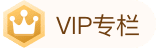
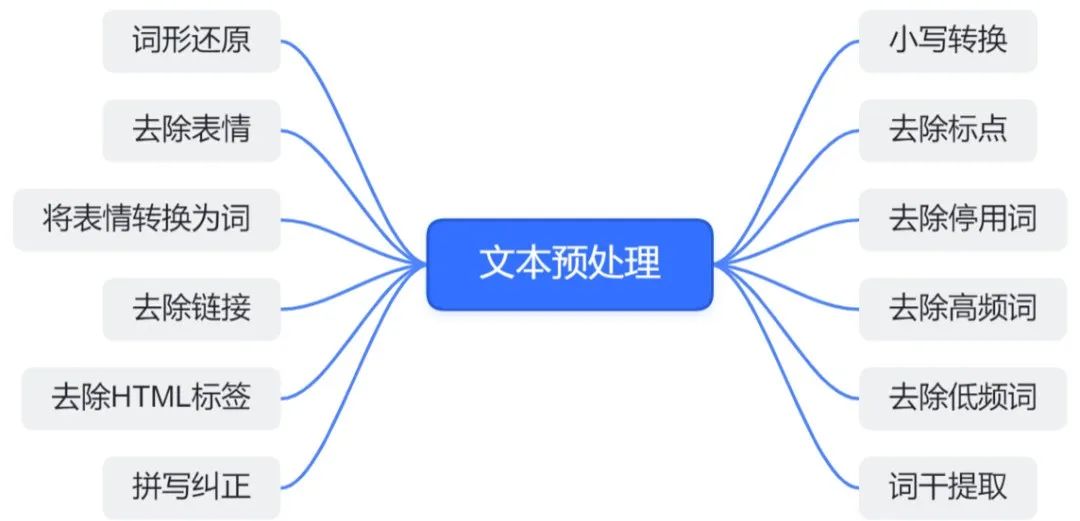
# 1. Python enumerate函数简介
Python 的 `enumerate()` 函数是一个内置函数,用于遍历序列中的元素,同时返回元素的索引和值。其语法如下:
```python
enumerate(iterable, start=0)
```
其中:
* `iterable`:要遍历的序列,可以是列表、元组、字符串或其他可迭代对象。
* `start`(可选):指定遍历的起始索引,默认为 0。
# 2. enumerate函数在自然语言处理中的应用
### 2.1 遍历文本数据
enumerate函数在自然语言处理中的一大应用是遍历文本数据。它可以逐个字符、逐个单词或逐个句子遍历文本,从而方便地对文本进行处理。
#### 2.1.1 逐个字符遍历
```python
text = "Hello, world!"
for index, char in enumerate(text):
print(f"Index: {index}, Character: {char}")
```
输出:
```
Index: 0, Character: H
Index: 1, Character: e
Index: 2, Character: l
Index: 3, Character: l
Index: 4, Character: o
Index: 5, Character: ,
Index: 6, Character:
Index: 7, Character: w
Index: 8, Character: o
Index: 9, Character: r
Index: 10, Character: l
Index: 11, Character: d
Index: 12, Character: !
```
#### 2.1.2 逐个单词遍历
```python
text = "This is a sample sentence."
for index, word in enumerate(text.split()):
print(f"Index: {index}, Word: {word}")
```
输出:
```
Index: 0, Word: This
Index: 1, Word: is
Index: 2, Word: a
Index: 3, Word: sample
Index: 4, Word: sentence
```
#### 2.1.3 逐个句子遍历
```python
text = "This is the first sentence. This is the second sentence."
for index, sentence in enumerate(text.split(".")):
print(f"Index: {index}, Sentence: {sentence}")
```
输出:
```
Index: 0, Sentence: This is the first sentence
Index: 1, Sentence: This is the second sentence
```
### 2.2 提升文本处理效率
enumerate函数还可以提升文本处理效率,因为它可以避免使用额外的变量来跟踪索引。这在处理大型文本数据集时尤其有用。
#### 2.2.1 优化文本清洗过程
```python
def clean_text(text):
cleaned_text = []
for index, char in enumerate(text):
if char.isalpha() or char.isspace():
cleaned_text.append(char)
return "".join(cleaned_text)
```
#### 2.2.2 加速特征工程
```python
def extract_features(text):
features = []
for index, word in enumerate(text.split()):
features.append(len(word))
return features
```
#### 2.2.3 提高模型训练速度
```python
def train_model(data):
X = []
y = []
for index, row in enumerate(data):
X.append(row[0])
y.append(row[1])
model.fit(X, y)
```
# 3. enumerate函数在自然语言处理中的实践案例
### 3.1 文本分词
文本分词是自然语言处理中的基本任务,它将文本分解为一个个独立的词语或符号。enumerate函数在文本分词中扮演着重要的角色,因为它可以帮助我们遍历文本中的每个字符或单词,并对其进行处理。
#### 3.1.1 基于空格的分词
最简单的文本分词方法是基于空格进行分词。我们可以使用enumerate函数遍历文本中的每个字符,并检查当前字符是否为空格。如果当前字符为空格,则表示一个单词的结束,我们可以将前一个字符组成的字符串作为分词结果。
```python
def tokenize_by_space(text):
"""基于空格对文本进行分词
Args:
text (str): 待分词的文本
Returns:
list[str]: 分词后的单词列表
"""
words = []
for i, char in enumerate(text):
if char == " ":
words.append(text[i-1])
return words
```
**代码逻辑分析:**
* 遍历文本中的每个字符,并获取其索引 `i` 和字符 `char`。
* 检查当前字符 `char` 是否为空格。
* 如果当前字符为空格,则表示一个单词的结束,将前一个字符组成的字符串 `text[i-1]` 添加到 `words` 列表中。
* 返回分词后的单词列表 `words`。
#### 3.1.2 基于正则表达式的分词
正则表达式是一种强大的模式匹配工具,它可以帮助我们匹配文本中符合特定模式的单词或符号。我们可以使用正则表达式来定义分词规则,然后使用enumerate函数遍历文本中的每个字符,并检查当前字符是否匹配分词规则。
```python
import re
def tokenize_by_regex(text, pattern):
"""基于正则表达式对文本进行分词
Args:
text (str): 待分词的文本
pattern (str): 分词规则的正则表达式
Returns:
list[str]: 分词后的单词列表
"""
words = []
for i, ch
```
0
0
相关推荐
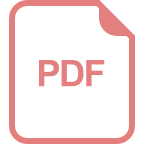
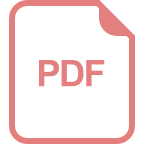
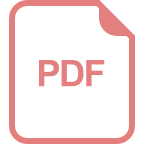
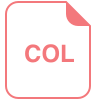
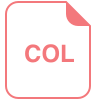
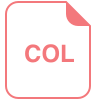
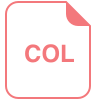

