设计一个学生类,包括学号姓名成绩计算学生的平均成绩,包括构造和析构函数
时间: 2023-06-23 19:08:28 浏览: 259
以下是一个简单的学生类的实现,包括学号、姓名、成绩和计算平均成绩的功能:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string stuID;
string name;
double score;
public:
Student(string id, string n, double s) : stuID(id), name(n), score(s) {} // 构造函数
~Student() {} // 析构函数
double getScore() const {
return score;
}
void display() const {
cout << "学号:" << stuID << endl;
cout << "姓名:" << name << endl;
cout << "成绩:" << score << endl;
}
static double calculateAverageScore(Student* students, int num) { // 静态成员函数,用于计算平均成绩
double sum = 0.0;
for (int i = 0; i < num; i++) {
sum += students[i].getScore();
}
return sum / num;
}
};
int main() {
Student s1("001", "张三", 90.5);
s1.display();
Student s2("002", "李四", 85.0);
s2.display();
Student s3("003", "王五", 78.5);
s3.display();
Student students[] = { s1, s2, s3 };
double averageScore = Student::calculateAverageScore(students, 3);
cout << "平均成绩:" << averageScore << endl;
return 0;
}
```
上述代码定义了一个学生类,其中包含了学号、姓名、成绩等属性,以及构造和析构函数。在 main 函数中,我们创建了三个学生对象,并将它们存储在一个数组中,然后调用了 calculateAverageScore 静态成员函数,计算出了所有学生的平均成绩。
阅读全文
相关推荐

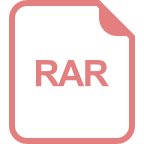















