设计一个学生类 包括学号姓名成绩计算学生的平均成绩包括构造函数和析构函数C++
时间: 2024-10-17 09:11:42 浏览: 24
在C++中设计一个简单的`Student`类,你可以按照下面的方式来实现:
```cpp
#include <iostream>
using namespace std;
class Student {
private:
int studentId;
string name;
double score; // 成绩
public:
// 构造函数 (Constructor)
Student(int id, const string& name) {
this->studentId = id;
this->name = name;
this->score = 0.0; // 初始化默认值
}
// 计算平均成绩 (Method for calculating average score)
double calculateAverageScore(double newScore) {
if (newScore >= 0.0) {
this->score += newScore;
return this->score / ++countOfScores(); // 假设有一个内部计数器countOfScores用于记录分数次数
} else {
cout << "Invalid score!" << endl;
return 0.0;
}
}
// 获取当前平均成绩
double getAverageScore() {
if (countOfScores()) {
return this->score / countOfScores();
} else {
return 0.0;
}
}
// 析构函数 (Destructor)
~Student() {
cout << "Student object with ID: " << studentId << " is destructed." << endl;
}
private:
int countOfScores() { // 这里只是一个简化的示例,实际应用中可能需要更复杂的数据结构来跟踪分数
static int counter = 0;
return counter++;
}
};
int main() {
Student student1(1, "张三");
student1.calculateAverageScore(95);
student1.calculateAverageScore(87);
cout << "Student " << student1.name << " 的平均成绩是: " << student1.getAverageScore() << endl;
return 0;
}
```
在这个例子中,我们创建了一个`Student`类,包含了学号、姓名和成绩属性,以及构造函数、计算平均成绩的方法和析构函数。注意,`countOfScores`是一个辅助函数,仅作为示例说明如何在没有外部数据管理的情况下简单地维护分数次数。
阅读全文
相关推荐
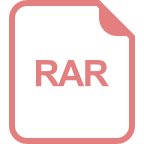
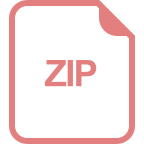
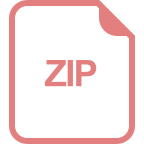















