html圣诞树源代码
时间: 2024-12-26 10:14:36 浏览: 5
### HTML Christmas Tree Source Code Example
To create an HTML-based Christmas tree with decorations such as ornaments using WebGL via Three.js, one can adapt the provided branch addition function to include spherical geometries representing balls or other decorative items on branches of a virtual Christmas tree[^1]. Below is an illustrative example that demonstrates how this might be achieved:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Christmas Tree</title>
<style>
body { margin: 0; }
canvas { display: block; }
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script>
// Set up scene and camera.
const scene = new THREE.Scene();
scene.background = new THREE.Color('black');
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.z = 5;
// Renderer setup.
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
function addStar() {
const geometry = new THREE.SphereGeometry(0.1, 32, 32);
const material = new THREE.MeshBasicMaterial({
color: 'gold',
wireframe: false
});
const star = new THREE.Mesh(geometry, material);
star.position.set(0, 4, 0); // Position at top of tree
scene.add(star);
}
function addOrnaments(points) {
points.forEach(point => {
let sphereGeom = new THREE.SphereGeometry(.05, 32, 32),
ornamentMat = new THREE.MeshPhongMaterial({
color: Math.random() * 0xffffff,
specular: 0x050505,
shininess: 100
}),
ornamentMesh = new THREE.Mesh(sphereGeom, ornamentMat);
ornamentMesh.position.copy(point);
scene.add(ornamentMesh);
});
}
addStar();
for (let i = 0; i < 10; ++i){
let angle = Math.PI * 2 * i / 10;
let length = 2 + Math.random(); // Random lengths for varied positions
let pointOnBranch = new THREE.Vector2(Math.cos(angle) * length, Math.sin(angle) * length);
// Add decoration near end of each branch
addOrnaments([pointOnBranch]);
}
animate();
function animate(){
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
</script>
</body>
</html>
```
This script initializes a simple web page displaying a black background with a gold star positioned atop what would represent the peak of a Christmas tree. Ornaments are added along calculated paths meant to simulate branches extending from the trunk upwards and outwards.
阅读全文
相关推荐
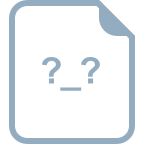

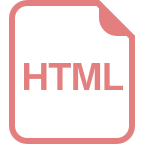
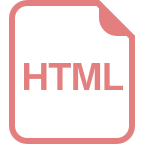
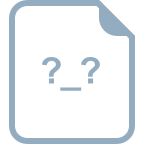
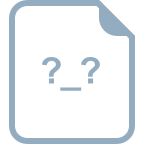
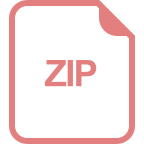
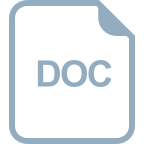
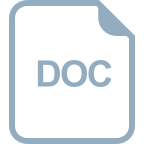
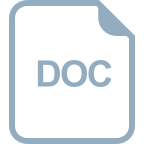


