m个盒子,有长宽高,堆盒子要求下面的盒子长宽高都大于上面的盒子,不包含等于。高度为堆出盒子的总高度,输出堆出盒子的最高高度。Java代码怎么写
时间: 2024-09-12 09:03:42 浏览: 62
这个问题可以通过使用动态规划(Dynamic Programming, DP)的方法来解决。首先,我们需要对盒子按照长宽高进行排序,按照长、宽、高的顺序来确保每个盒子的长、宽和高都大于它下面的盒子。
以下是一个可能的Java代码实现步骤:
1. 首先定义一个盒子类(Box),包含长、宽、高三个属性,并重写比较方法,以便可以根据长宽高对盒子进行排序。
2. 使用排序算法对盒子数组进行排序。
3. 使用动态规划的方法来计算最大堆叠高度。创建一个数组dp,其中dp[i]表示以第i个盒子为顶部时能够堆叠的最大高度。
4. 遍历每个盒子,对于每个盒子,查找所有比它小的盒子,并更新dp[i]为所有可能的堆叠方式中的最大高度。
5. 最后,遍历dp数组找出最大值即为所求。
以下是代码示例:
```java
class Box {
int l, w, h;
public Box(int l, int w, int h) {
this.l = l;
this.w = w;
this.h = h;
}
}
public class StackBoxes {
public int pileBoxes(int[][] boxes) {
Box[] boxArray = new Box[boxes.length];
for (int i = 0; i < boxes.length; i++) {
boxArray[i] = new Box(boxes[i][0], boxes[i][1], boxes[i][2]);
}
// Sort the boxes
Arrays.sort(boxArray, new Comparator<Box>() {
@Override
public int compare(Box b1, Box b2) {
if (b1.l == b2.l) {
if (b1.w == b2.w) {
return Integer.compare(b1.h, b2.h);
} else {
return Integer.compare(b1.w, b2.w);
}
} else {
return Integer.compare(b1.l, b2.l);
}
}
});
// Dynamic programming array
int[] dp = new int[boxes.length];
int maxHeight = 0;
for (int i = 0; i < boxArray.length; i++) {
dp[i] = boxArray[i].h;
for (int j = 0; j < i; j++) {
if (boxArray[i].l > boxArray[j].l && boxArray[i].w > boxArray[j].w && boxArray[i].h > boxArray[j].h) {
dp[i] = Math.max(dp[i], dp[j] + boxArray[i].h);
}
}
maxHeight = Math.max(maxHeight, dp[i]);
}
return maxHeight;
}
}
```
阅读全文
相关推荐

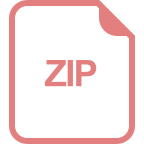
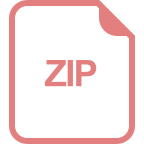
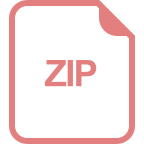
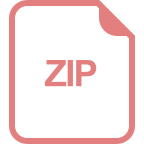
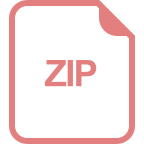
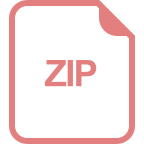
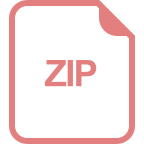
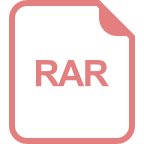
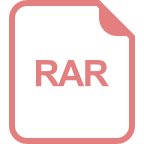
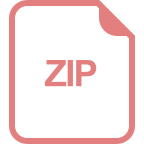
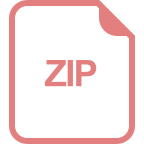
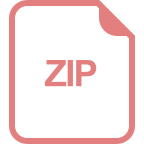
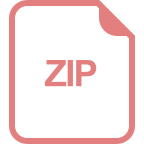
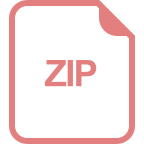