m个盒子,每行输入3个数字表示长宽高,堆盒子要求下面的盒子长宽高都大于上面的盒子,不包含等于。高度为堆出盒子的总高度,输出堆出盒子的最高高度。Java代码怎么写
时间: 2024-09-12 12:03:43 浏览: 65
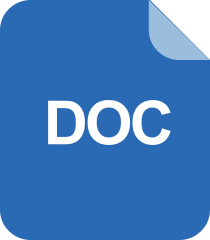
长方体正方体复习(提高).doc
要实现这样一个功能,我们可以使用贪心算法来解决问题。基本思路是先对输入的盒子按照长、宽、高进行排序,使得较小的盒子位于较大的盒子下面,然后遍历排序后的盒子,每次找到可以堆叠在其上面的盒子,累加高度并更新最大高度。下面是使用Java实现该算法的示例代码:
```java
import java.util.Scanner;
import java.util.Arrays;
public class BoxStacking {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int m = scanner.nextInt(); // 输入盒子的数量
Box[] boxes = new Box[m];
for (int i = 0; i < m; i++) {
int length = scanner.nextInt();
int width = scanner.nextInt();
int height = scanner.nextInt();
boxes[i] = new Box(length, width, height);
}
scanner.close();
// 按照长、宽、高排序
Arrays.sort(boxes, (a, b) -> {
if (a.length != b.length) return a.length - b.length;
if (a.width != b.width) return a.width - b.width;
return a.height - b.height;
});
// 寻找最高的堆叠高度
int maxHeight = 0;
int[] dp = new int[m];
Arrays.fill(dp, 0);
for (int i = 0; i < m; i++) {
dp[i] = boxes[i].height; // 初始化dp数组
for (int j = 0; j < i; j++) {
if (boxes[i].canStackOn(boxes[j])) {
dp[i] = Math.max(dp[i], dp[j] + boxes[i].height);
}
}
maxHeight = Math.max(maxHeight, dp[i]); // 更新最大高度
}
System.out.println(maxHeight); // 输出最大高度
}
}
class Box {
int length, width, height;
public Box(int length, int width, int height) {
this.length = length;
this.width = width;
this.height = height;
}
public boolean canStackOn(Box box) {
// 判断当前盒子是否可以堆叠在指定盒子之上
return box.length > this.length && box.width > this.width && box.height > this.height;
}
}
```
在这段代码中,`Box` 类用于表示盒子,包含长、宽、高三个属性以及一个判断是否可以堆叠在另一个盒子上的方法 `canStackOn`。主方法中首先读取盒子的数量和每个盒子的长宽高,然后根据长、宽、高进行排序。之后使用动态规划的方式计算能够堆叠的最大高度。
阅读全文
相关推荐
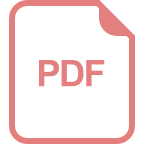
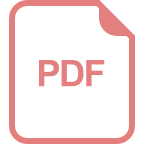

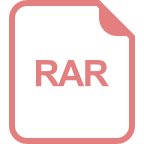
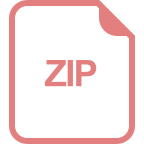
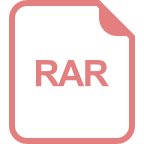
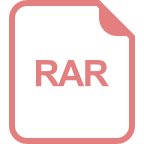
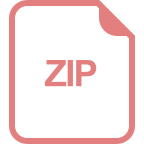
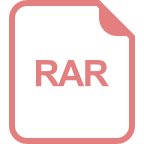
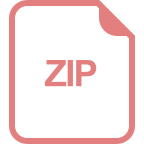
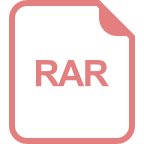
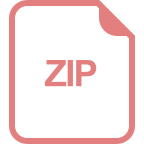
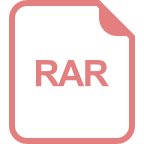