如何遍历map c++
时间: 2023-09-07 21:12:04 浏览: 42
在 C++ 中,可以使用迭代器来遍历 std::map。以下是一种常见的遍历方式:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
// 使用迭代器遍历map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
在上面的示例中,我们创建了一个包含整数作为键和字符串作为值的 std::map。然后,通过使用 begin() 和 end() 方法获取迭代器的起始和结束位置,并使用 auto 关键字简化迭代器类型的声明。在 for 循环中,我们使用迭代器 it 遍历整个 map,并输出每个键和值的内容。
希望这可以帮助你进行 map 的遍历!如有任何疑问,请随时提问。
相关问题
顺序遍历map c++
在C语言中,map通常是用结构体实现的,因此要遍历map,可以使用结构体指针和循环遍历的方式。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct map_node {
char* key;
int value;
struct map_node* next;
};
struct map {
struct map_node** buckets;
int num_buckets;
};
struct map* create_map(int num_buckets) {
struct map* map = malloc(sizeof(struct map));
map->buckets = calloc(num_buckets, sizeof(struct map_node*));
map->num_buckets = num_buckets;
return map;
}
void add_to_map(struct map* map, char* key, int value) {
int index = abs(hash(key)) % map->num_buckets;
struct map_node* node = map->buckets[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
node->value = value;
return;
}
node = node->next;
}
node = malloc(sizeof(struct map_node));
node->key = strdup(key);
node->value = value;
node->next = map->buckets[index];
map->buckets[index] = node;
}
int get_from_map(struct map* map, char* key) {
int index = abs(hash(key)) % map->num_buckets;
struct map_node* node = map->buckets[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
node = node->next;
}
return 0;
}
void free_map(struct map* map) {
for (int i = 0; i < map->num_buckets; i++) {
struct map_node* node = map->buckets[i];
while (node != NULL) {
struct map_node* next = node->next;
free(node->key);
free(node);
node = next;
}
}
free(map->buckets);
free(map);
}
int main() {
struct map* map = create_map(10);
add_to_map(map, "foo", 1);
add_to_map(map, "bar", 2);
add_to_map(map, "baz", 3);
for (int i = 0; i < map->num_buckets; i++) {
struct map_node* node = map->buckets[i];
while (node != NULL) {
printf("%s: %d\n", node->key, node->value);
node = node->next;
}
}
free_map(map);
return 0;
}
```
这个示例代码中,我们使用了一个简单的哈希函数来计算字符串的哈希值,然后用哈希值来确定在哪个桶中存储节点。添加节点时,如果发现已经存在相同的键,则更新节点的值。遍历map时,我们使用两层循环,外层循环遍历所有桶,内层循环遍历桶中的所有节点,并打印它们的键和值。最后,我们释放所有节点并销毁map。
c++ 遍历map
在C++中,遍历`std::map`可以使用迭代器来实现。`std::map`是一个关联容器,它按照键的顺序存储键值对,并且每个键只能出现一次。
以下是遍历`std::map`的示例代码:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
// 使用迭代器遍历map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
在上面的示例中,我们创建了一个`std::map`对象`myMap`,并向其中插入了三个键值对。然后,我们使用迭代器`it`遍历整个`myMap`,并输出每个键值对的键和值。
输出结果为:
```
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
相关推荐
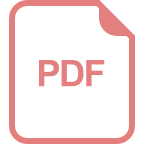
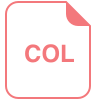











