基于python的使用surf特征图像的图像拼接
时间: 2023-12-29 20:03:13 浏览: 147
好的,我可以回答你的问题。
如果你想要使用Python来实现基于SURF特征的图像拼接,可以使用OpenCV库中的SURF算法和图像拼接函数。下面是一个简单的示例代码:
```python
import cv2
import numpy as np
# 读取要拼接的两张图片
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 创建SURF对象
surf = cv2.xfeatures2d.SURF_create()
# 在两张图片上分别提取关键点和特征描述符
kp1, des1 = surf.detectAndCompute(img1, None)
kp2, des2 = surf.detectAndCompute(img2, None)
# 匹配两张图片的特征点
bf = cv2.BFMatcher()
matches = bf.match(des1, des2)
# 将匹配到的特征点按照距离从小到大排序
matches = sorted(matches, key=lambda x: x.distance)
# 取出前10个匹配点
good_matches = matches[:10]
# 获取匹配点的坐标
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 计算透视变换矩阵
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 对img1进行透视变换,将其拼接到img2上
result = cv2.warpPerspective(img1, M, (img1.shape[1] + img2.shape[1], img2.shape[0]))
result[0:img2.shape[0], 0:img2.shape[1]] = img2
# 显示拼接结果
cv2.imshow('result', result)
cv2.waitKey(0)
```
需要注意的是,在实际应用中,可能需要对匹配点进行进一步的筛选和优化,以达到更好的拼接效果。
阅读全文
相关推荐
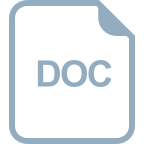
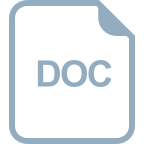
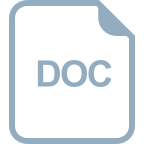
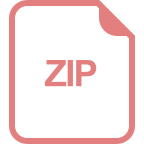
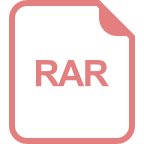
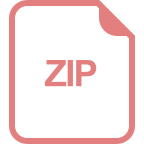
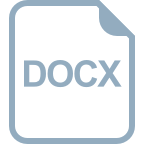
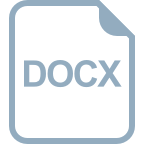
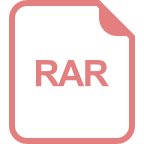
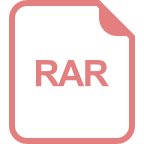
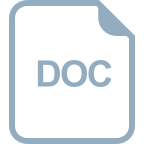




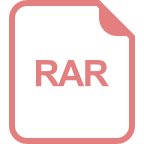
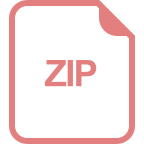
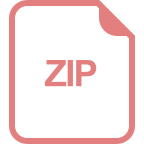
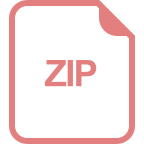