某班级学生的姓名、学号、成绩列表如下: Name Number Score Lei Zhenzi 101401 82 Jiang Ziya 100032 90 Ne Zha 101674 70 Shen Gongbao 101982 87 Jiu Weihu 107431 75 Tian Zun 100001 98 Tai Yi 101009 81 Yang Jian 101321 63 Huang Feihu 101567 72 Zhou Wang 108160 55 Li Jing 102456 84 Tu Xingsun 102245 65 内容: 1选择合适的数据结构,按上表序列,构造一棵完全二叉树,每个结点中保存学生的姓名、学号和成绩信息。①按后序(递归)遍历输出此二叉树;②按先序(非递归)算法出此二叉树;③按广度优先遍历算法输出此二叉树; 2按上表序列,构造一棵二叉排序树,每个结点中保存学生的姓名、学号和成绩信息。①按中序遍历输出此二叉树;②按广度优先遍历算法输出此二叉树;③以姓名为关键字,查找某人的成绩单信息;以Tai Yi为例输出查询结果;④如果想输出所有成绩低于或等于75的所有信息;该如何做?
时间: 2024-02-10 14:32:44 浏览: 82
1. 对于第一个问题,我们可以选择使用数组来存储班级学生的信息,然后根据学号的顺序构造一棵完全二叉树。对于每个节点,我们可以使用一个结构体来保存学生的姓名、学号和成绩信息。具体实现如下:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct Student {
string name;
int number;
int score;
};
void postOrderTraversal(Student* tree, int index, int n) {
if (index >= n) {
return;
}
postOrderTraversal(tree, 2 * index + 1, n);
postOrderTraversal(tree, 2 * index + 2, n);
cout << tree[index].name << " " << tree[index].number << " " << tree[index].score << endl;
}
void preOrderTraversal(Student* tree, int n) {
stack<int> s;
s.push(0);
while (!s.empty()) {
int index = s.top();
s.pop();
cout << tree[index].name << " " << tree[index].number << " " << tree[index].score << endl;
if (2 * index + 2 < n) {
s.push(2 * index + 2);
}
if (2 * index + 1 < n) {
s.push(2 * index + 1);
}
}
}
void bfs(Student* tree, int n) {
queue<int> q;
q.push(0);
while (!q.empty()) {
int index = q.front();
q.pop();
cout << tree[index].name << " " << tree[index].number << " " << tree[index].score << endl;
if (2 * index + 1 < n) {
q.push(2 * index + 1);
}
if (2 * index + 2 < n) {
q.push(2 * index + 2);
}
}
}
int main() {
Student tree[] = {
{"Lei Zhenzi", 101401, 82},
{"Jiang Ziya", 100032, 90},
{"Ne Zha", 101674, 70},
{"Shen Gongbao", 101982, 87},
{"Jiu Weihu", 107431, 75},
{"Tian Zun", 100001, 98},
{"Tai Yi", 101009, 81},
{"Yang Jian", 101321, 63},
{"Huang Feihu", 101567, 72},
{"Zhou Wang", 108160, 55},
{"Li Jing", 102456, 84},
{"Tu Xingsun", 102245, 65}
};
int n = sizeof(tree) / sizeof(tree[0]);
postOrderTraversal(tree, 0, n);
preOrderTraversal(tree, n);
bfs(tree, n);
return 0;
}
```
2. 对于第二个问题,我们可以使用二叉搜索树来存储班级学生的信息,具体实现如下:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct Student {
string name;
int number;
int score;
Student* left;
Student* right;
};
void inorderTraversal(Student* root) {
if (root == nullptr) {
return;
}
inorderTraversal(root->left);
cout << root->name << " " << root->number << " " << root->score << endl;
inorderTraversal(root->right);
}
void bfs(Student* root) {
queue<Student*> q;
q.push(root);
while (!q.empty()) {
Student* node = q.front();
q.pop();
cout << node->name << " " << node->number << " " << node->score << endl;
if (node->left != nullptr) {
q.push(node->left);
}
if (node->right != nullptr) {
q.push(node->right);
}
}
}
Student* search(Student* root, string name) {
if (root == nullptr || root->name == name) {
return root;
}
if (root->name < name) {
return search(root->right, name);
}
else {
return search(root->left, name);
}
}
void searchScore(Student* root, int score) {
if (root == nullptr) {
return;
}
if (root->score <= score) {
cout << root->name << " " << root->number << " " << root->score << endl;
}
searchScore(root->left, score);
searchScore(root->right, score);
}
int main() {
Student* root = nullptr;
root = new Student{"Lei Zhenzi", 101401, 82, nullptr, nullptr};
root->left = new Student{"Jiang Ziya", 100032, 90, nullptr, nullptr};
root->right = new Student{"Ne Zha", 101674, 70, nullptr, nullptr};
root->left->left = new Student{"Shen Gongbao", 101982, 87, nullptr, nullptr};
root->left->right = new Student{"Jiu Weihu", 107431, 75, nullptr, nullptr};
root->right->left = new Student{"Tian Zun", 100001, 98, nullptr, nullptr};
root->right->right = new Student{"Tai Yi", 101009, 81, nullptr, nullptr};
root->left->left->left = new Student{"Yang Jian", 101321, 63, nullptr, nullptr};
root->left->left->right = new Student{"Huang Feihu", 101567, 72, nullptr, nullptr};
root->right->left->left = new Student{"Zhou Wang", 108160, 55, nullptr, nullptr};
root->right->right->right = new Student{"Li Jing", 102456, 84, nullptr, nullptr};
root->right->right->left = new Student{"Tu Xingsun", 102245, 65, nullptr, nullptr};
inorderTraversal(root);
bfs(root);
Student* student = search(root, "Tai Yi");
cout << student->name << " " << student->number << " " << student->score << endl;
searchScore(root, 75);
return 0;
}
```
以上两个问题的代码都是在 C++ 下编写,你可以根据需要进行修改。
阅读全文
相关推荐

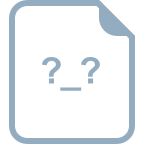
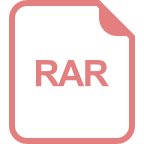
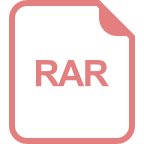
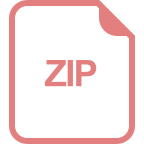
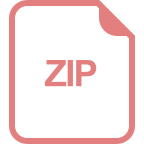