netty 实现代理服务器
时间: 2023-08-23 09:07:49 浏览: 82
Netty是一款基于NIO的网络编程框架,提供了高效、稳定、灵活的网络编程能力。使用Netty实现代理服务器可以简化开发过程,提高性能和可维护性。
以下是使用Netty实现代理服务器的示例代码:
```
import io.netty.bootstrap.Bootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.channel.*;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.http.*;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import io.netty.handler.stream.ChunkedWriteHandler;
public class ProxyServer {
public static void main(String[] args) throws Exception {
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(workerGroup)
.channel(NioSocketChannel.class)
.handler(new LoggingHandler(LogLevel.INFO))
.option(ChannelOption.AUTO_READ, false)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new HttpClientCodec());
ch.pipeline().addLast(new HttpObjectAggregator(65536));
ch.pipeline().addLast(new ChunkedWriteHandler());
ch.pipeline().addLast(new ProxyServerHandler());
}
});
ChannelFuture future = bootstrap.connect("www.example.com", 80).sync();
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
}
}
private static class ProxyServerHandler extends ChannelInboundHandlerAdapter {
private Channel remoteChannel;
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
remoteChannel = ctx.channel();
ctx.read();
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof HttpRequest) {
HttpRequest request = (HttpRequest) msg;
String host = request.headers().get("Host");
ChannelFuture future = new Bootstrap()
.group(ctx.channel().eventLoop())
.channel(ctx.channel().getClass())
.handler(new LoggingHandler(LogLevel.INFO))
.option(ChannelOption.AUTO_READ, false)
.option(ChannelOption.CONNECT_TIMEOUT_MILLIS, 10000)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new HttpResponseDecoder());
ch.pipeline().addLast(new HttpObjectAggregator(65536));
ch.pipeline().addLast(new ChunkedWriteHandler());
ch.pipeline().addLast(new ChannelInboundHandlerAdapter() {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
ctx.writeAndFlush(request);
ctx.read();
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof HttpResponse) {
HttpResponse response = (HttpResponse) msg;
response.headers().remove("Transfer-Encoding");
response.headers().remove("Content-Length");
remoteChannel.writeAndFlush(response);
remoteChannel.writeAndFlush(new ChunkedNioStream((ByteBuf) msg));
} else if (msg instanceof HttpContent) {
remoteChannel.writeAndFlush(new ChunkedNioStream((ByteBuf) msg));
if (msg instanceof LastHttpContent) {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
}
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
});
}
})
.connect(host, 80);
remoteChannel.config().setAutoRead(false);
future.addListener((ChannelFutureListener) future1 -> {
if (future1.isSuccess()) {
remoteChannel.config().setAutoRead(true);
ctx.channel().config().setAutoRead(true);
} else {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
ctx.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
});
} else if (msg instanceof HttpContent) {
remoteChannel.writeAndFlush(new ChunkedNioStream((ByteBuf) msg));
if (msg instanceof LastHttpContent) {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT);
}
}
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
if (remoteChannel != null) {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
if (remoteChannel != null) {
remoteChannel.writeAndFlush(LastHttpContent.EMPTY_LAST_CONTENT)
.addListener(ChannelFutureListener.CLOSE);
}
ctx.close();
}
}
}
```
以上代码中,代理服务器连接到目标服务器的IP地址和端口号是硬编码的,你需要根据实际情况进行修改。在启动代理服务器之后,当客户端发送HTTP请求时,会在一个新的线程中处理请求,解析请求并连接到目标服务器,将请求转发给目标服务器。接收到目标服务器的响应后,将响应转发给客户端。
相关推荐
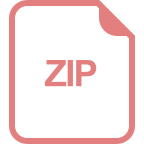
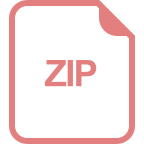














