用netty框架写一个socks代理
时间: 2023-05-17 09:04:43 浏览: 104
好的,以下是使用 Netty 框架编写 SOCKS 代理的示例代码:
```
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.socksx.v5.DefaultSocks5CommandRequest;
import io.netty.handler.codec.socksx.v5.DefaultSocks5InitialRequest;
import io.netty.handler.codec.socksx.v5.Socks5AddressType;
import io.netty.handler.codec.socksx.v5.Socks5ClientEncoder;
import io.netty.handler.codec.socksx.v5.Socks5CommandRequest;
import io.netty.handler.codec.socksx.v5.Socks5CommandResponse;
import io.netty.handler.codec.socksx.v5.Socks5InitialRequest;
import io.netty.handler.codec.socksx.v5.Socks5InitialResponse;
import io.netty.handler.codec.socksx.v5.Socks5PasswordAuthRequest;
import io.netty.handler.codec.socksx.v5.Socks5PasswordAuthResponse;
import io.netty.handler.codec.socksx.v5.Socks5ServerEncoder;
import io.netty.handler.codec.socksx.v5.Socks5UserPasswordAuthRequest;
import io.netty.handler.codec.socksx.v5.Socks5UserPasswordAuthResponse;
import io.netty.util.CharsetUtil;
public class SocksProxyClient {
private static final String SOCKS_HOST = "127.0.0.1";
private static final int SOCKS_PORT = 1080;
private static final String REMOTE_HOST = "www.google.com";
private static final int REMOTE_PORT = 80;
public static void main(String[] args) throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new Socks5ClientEncoder(Socks5AddressType.DOMAIN));
ch.pipeline().addLast(new SocksProxyClientHandler());
}
});
ChannelFuture f = b.connect(SOCKS_HOST, SOCKS_PORT).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
private static class SocksProxyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// Send initial SOCKS5 handshake request
ctx.writeAndFlush(new DefaultSocks5InitialRequest(Socks5AuthMethod.NO_AUTH));
// Send SOCKS5 command request to connect to remote host
Socks5CommandRequest cmdRequest = new DefaultSocks5CommandRequest(
Socks5CommandRequest.CmdType.CONNECT,
Socks5AddressType.DOMAIN,
REMOTE_HOST,
REMOTE_PORT);
ctx.writeAndFlush(cmdRequest);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof Socks5InitialResponse) {
// Handle SOCKS5 initial response
Socks5InitialResponse initResponse = (Socks5InitialResponse) msg;
if (initResponse.authMethod() == Socks5AuthMethod.PASSWORD) {
// Send SOCKS5 username/password authentication request
ctx.writeAndFlush(new Socks5PasswordAuthRequest("username", "password"));
}
} else if (msg instanceof Socks5PasswordAuthResponse) {
// Handle SOCKS5 username/password authentication response
Socks5PasswordAuthResponse authResponse = (Socks5PasswordAuthResponse) msg;
if (authResponse.status() == Socks5PasswordAuthStatus.SUCCESS) {
// Send SOCKS5 command request to connect to remote host
Socks5CommandRequest cmdRequest = new DefaultSocks5CommandRequest(
Socks5CommandRequest.CmdType.CONNECT,
Socks5AddressType.DOMAIN,
REMOTE_HOST,
REMOTE_PORT);
ctx.writeAndFlush(cmdRequest);
}
} else if (msg instanceof Socks5CommandResponse) {
// Handle SOCKS5 command response
Socks5CommandResponse cmdResponse = (Socks5CommandResponse) msg;
if (cmdResponse.status() == Socks5CommandStatus.SUCCESS) {
// Send HTTP request to remote host
Channel remoteChannel = ctx.channel();
remoteChannel.pipeline().remove(Socks5ServerEncoder.class);
remoteChannel.pipeline().addLast(new HttpProxyClientHandler());
String httpRequest = "GET / HTTP/1.1\r\n" +
"Host: " + REMOTE_HOST + "\r\n" +
"Connection: close\r\n\r\n";
remoteChannel.writeAndFlush(httpRequest);
}
} else {
// Handle HTTP response from remote host
String httpResponse = (String) msg;
System.out.println(httpResponse);
ctx.close();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
private static class HttpProxyClientHandler extends ChannelInboundHandlerAdapter {
private StringBuilder responseBuilder = new StringBuilder();
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
String httpResponse = (String) msg;
responseBuilder.append(httpResponse);
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
String httpResponse = responseBuilder.toString();
System.out.println(httpResponse);
ctx.close();
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
}
```
这个示例代码使用 Netty 框架实现了一个 SOCKS5 代理客户端,可以连接到指定的 SOCKS5 代理服务器,并通过代理服务器连接到指定的远程主机。在连接到远程主机后,它会发送一个 HTTP GET 请求,并打印出远程主机返回的 HTTP 响应。
相关推荐
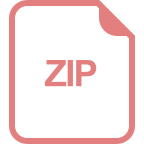
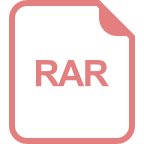
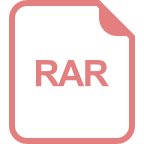














