用netty框架写一个socks代理,输出日志使用基于log4j的slf4j,不要使用system.out
时间: 2023-05-17 11:04:46 浏览: 68
好的,这是一个编程类的问题。使用Netty框架编写Socks代理并输出日志,可以使用基于Log4j的Slf4j,而不是System.out。以下是示例代码:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.socksx.SocksAddressType;
import io.netty.handler.codec.socksx.SocksCmdRequest;
import io.netty.handler.codec.socksx.SocksCmdResponse;
import io.netty.handler.codec.socksx.SocksCmdType;
import io.netty.handler.codec.socksx.SocksInitRequest;
import io.netty.handler.codec.socksx.SocksInitResponse;
import io.netty.handler.codec.socksx.SocksMessage;
import io.netty.handler.codec.socksx.SocksProtocolVersion;
import io.netty.handler.codec.socksx.SocksResponse;
import io.netty.handler.codec.socksx.v5.DefaultSocks5CommandRequest;
import io.netty.handler.codec.socksx.v5.Socks5ClientEncoder;
import io.netty.handler.codec.socksx.v5.Socks5CommandRequest;
import io.netty.handler.codec.socksx.v5.Socks5CommandResponseDecoder;
import io.netty.handler.codec.socksx.v5.Socks5InitialRequest;
import io.netty.handler.codec.socksx.v5.Socks5InitialResponseDecoder;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class SocksProxyClient {
private static final Logger logger = LoggerFactory.getLogger(SocksProxyClient.class);
private final String proxyHost;
private final int proxyPort;
private final String targetHost;
private final int targetPort;
public SocksProxyClient(String proxyHost, int proxyPort, String targetHost, int targetPort) {
this.proxyHost = proxyHost;
this.proxyPort = proxyPort;
this.targetHost = targetHost;
this.targetPort = targetPort;
}
public void start() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new LoggingHandler(LogLevel.INFO))
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline p = ch.pipeline();
p.addLast(new Socks5InitialResponseDecoder());
p.addLast(new Socks5CommandResponseDecoder());
p.addLast(new Socks5ClientEncoder());
p.addLast(new SocksProxyClientHandler());
}
});
ChannelFuture f = b.connect(proxyHost, proxyPort).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
private class SocksProxyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
Socks5InitialRequest initialRequest = new DefaultSocks5InitialRequest(SocksProtocolVersion.SOCKS5);
ctx.writeAndFlush(initialRequest);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof Socks5InitialResponse) {
Socks5InitialResponse initialResponse = (Socks5InitialResponse) msg;
if (initialResponse.authMethods().contains(Socks5AuthMethod.NO_AUTH)) {
Socks5CommandRequest commandRequest = new DefaultSocks5CommandRequest(Socks5CommandType.CONNECT, SocksAddressType.DOMAIN, targetHost, targetPort);
ctx.writeAndFlush(commandRequest);
} else {
logger.error("Socks5 proxy authentication not supported");
ctx.close();
}
} else if (msg instanceof Socks5CommandResponse) {
Socks5CommandResponse commandResponse = (Socks5CommandResponse) msg;
if (commandResponse.status().equals(Socks5CommandStatus.SUCCESS)) {
logger.info("Socks5 proxy connection established");
// TODO: handle proxy connection
} else {
logger.error("Socks5 proxy connection failed: {}", commandResponse.status());
ctx.close();
}
} else {
logger.error("Unexpected message type: {}", msg.getClass().getName());
ctx.close();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
logger.error("Socks proxy client error", cause);
ctx.close();
}
}
public static void main(String[] args) throws Exception {
String proxyHost = "127.0.0.1";
int proxyPort = 1080;
String targetHost = "www.baidu.com";
int targetPort = 80;
SocksProxyClient client = new SocksProxyClient(proxyHost, proxyPort, targetHost, targetPort);
client.start();
}
}
```
这是一个简单的Socks代理客户端,它使用Netty框架和Slf4j记录日志。在这个示例中,我们连接到一个Socks5代理服务器,并尝试连接到目标主机和端口。如果连接成功,我们可以在TODO部分处理代理连接。如果连接失败,我们将关闭连接并记录错误日志。
相关推荐
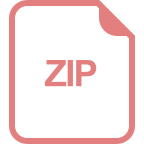
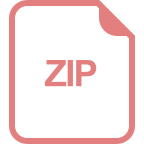














