用python实现基于梯度下降法对表格的“房屋单价”属性建立逻辑斯蒂分类模型代码
时间: 2024-04-29 11:26:12 浏览: 8
请问您的数据集已经处理好并且包含房屋单价和其他必要变量吗?如果是的话,您可以按照以下步骤使用Python实现基于梯度下降法对表格的“房屋单价”属性建立逻辑斯蒂分类模型。
1. 导入必要的包和数据集
```
import pandas as pd
import numpy as np
data = pd.read_csv("your_data.csv")
```
2. 预处理数据
对数据进行必要的预处理,包括去掉缺失值,转换categorical变量为dummy variables,将数据分为training set和testing set。假设房屋单价是“price”,您可以进行如下预处理步骤。
```
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
# 去除缺失值
data.dropna(inplace=True)
# 将categorical变量转为dummy variables
cat_vars = ['neighborhood', 'property_type', 'built_year']
for var in cat_vars:
cat_list = pd.get_dummies(data[var], prefix=var)
data = data.join(cat_list)
# 将价格标签编码为0和1
le = LabelEncoder()
data['price'] = le.fit_transform(data['price'])
# 将数据分为training set和testing set
X = data.drop('price', axis=1)
y = data['price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
3. 定义逻辑斯蒂分类模型
```
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def hypothesis(X, theta):
z = np.dot(X, theta)
return sigmoid(z)
def cost(X, y, theta):
m = len(y)
h = hypothesis(X, theta)
J = -1/m * (np.dot(y, np.log(h)) + np.dot((1-y), np.log(1-h)))
return J
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = []
for i in range(num_iters):
h = hypothesis(X, theta)
theta = theta - alpha/m * np.dot(X.T, (h-y))
J_history.append(cost(X, y, theta))
return theta, J_history
```
4. 训练模型
使用gradient_descent函数训练逻辑斯蒂分类模型,在每次迭代中计算代价函数J。
```
n = X_train.shape[1]
theta = np.zeros(n)
alpha = 0.01
num_iters = 1000
theta_final, J_history = gradient_descent(X_train, y_train, theta, alpha, num_iters)
```
5. 评估模型
使用计算得出的theta_final对testing set进行预测,并计算分类准确率。
```
def predict(X, theta):
h = hypothesis(X, theta)
y_pred = np.array([1 if i >= 0.5 else 0 for i in h])
return y_pred
y_pred_test = predict(X_test, theta_final)
from sklearn.metrics import accuracy_score
print(accuracy_score(y_test, y_pred_test))
```
以上是一个基于梯度下降法的逻辑斯蒂分类模型的Python代码实现,您可以根据自己的数据集和需求进行对应的修改和调整。
相关推荐
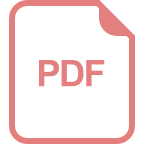














