用Java写一个快速排序
时间: 2024-05-02 14:20:56 浏览: 57
以下是用Java编写的快速排序算法:
```java
public class QuickSort {
public static void main(String[] args) {
int[] arr = {10, 7, 8, 9, 1, 5};
int n = arr.length;
quickSort(arr, 0, n - 1);
System.out.println("排序后的数组:");
for (int i : arr) {
System.out.print(i + " ");
}
}
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
}
```
在这个例子中,我们使用了一个递归函数quickSort()来对数组进行排序。这个函数采用了快速排序算法的核心思想——将一个数组划分为两个子数组,并对每个子数组进行递归排序。在每一次递归中,我们选择一个基准元素pivot,并将其放置在合适的位置,使得其左边的所有元素都小于它,右边的所有元素都大于它。这个过程称为分区。
我们使用一个分区函数partition()来实现分区。在这个函数中,我们选择数组的最后一个元素作为基准元素pivot,并从数组的第一个元素开始遍历数组。对于每个元素,如果它小于基准元素pivot,我们就将其与数组中的第i+1个元素交换位置,i的初始值为low-1。这样,所有小于pivot的元素都会被放置在数组的左边,而所有大于pivot的元素都会被放置在数组的右边。最后,我们将基准元素pivot与数组中的第i+1个元素交换位置,这样基准元素就被放置在了正确的位置。
通过不断递归调用quickSort()函数,我们最终可以将整个数组排序。快速排序算法的时间复杂度为O(nlogn)。
阅读全文
相关推荐
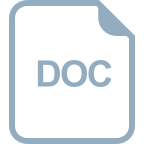
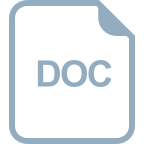
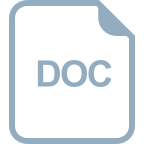
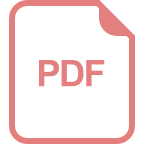
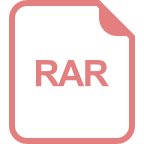
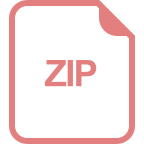
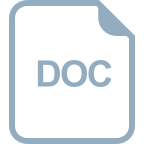
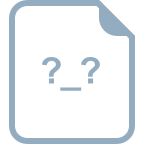
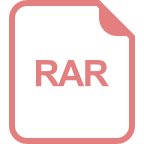
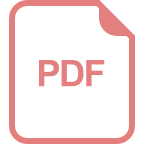
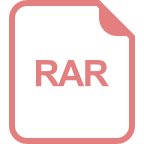
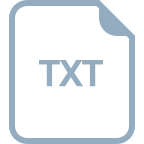
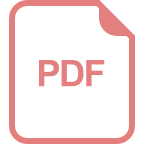
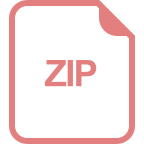
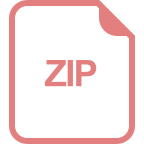