c#如何通过属性名称从自定义类中获取属性对象
时间: 2024-03-02 11:49:14 浏览: 71
在C#中,可以使用反射来通过属性名称从自定义类中获取属性对象。下面是一个示例:
```csharp
using System;
using System.Reflection;
class MyClass
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main(string[] args)
{
MyClass myObject = new MyClass();
myObject.Name = "John";
myObject.Age = 30;
PropertyInfo nameProperty = myObject.GetType().GetProperty("Name");
PropertyInfo ageProperty = myObject.GetType().GetProperty("Age");
Console.WriteLine("Name: {0}", nameProperty.GetValue(myObject));
Console.WriteLine("Age: {0}", ageProperty.GetValue(myObject));
}
}
```
在上面的示例中,`MyClass`类包含名为`Name`和`Age`的属性。在`Main`方法中,我们创建了一个`MyClass`对象并设置了`Name`和`Age`属性的值。然后,我们使用`GetType`方法来获取`MyClass`类型的`Type`对象。使用`GetProperty`方法,我们可以从`Type`对象中获取`Name`和`Age`属性的`PropertyInfo`对象。最后,我们使用`GetValue`方法从`myObject`对象中获取`Name`和`Age`属性的值。
需要注意的是,这种实现方式需要使用反射,因此可能会对性能产生影响。并且需要确保属性名称拼写正确,否则会引发`NullReferenceException`异常。
阅读全文
相关推荐
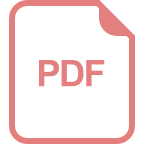
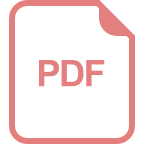
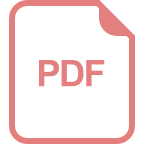















