用混合遗传模拟退火算法求解TSP问题并给出实现代码
时间: 2024-02-11 07:07:01 浏览: 30
好的,以下是用混合遗传模拟退火算法求解TSP问题的实现代码:
```python
import numpy as np
import random
# 计算路径长度
def calc_path_len(path, dist_mat):
length = 0
for i in range(len(path) - 1):
length += dist_mat[path[i]][path[i+1]]
length += dist_mat[path[-1]][path[0]]
return length
# 初始化种群
def init_population(pop_size, num_cities):
population = []
for i in range(pop_size):
path = list(range(num_cities))
random.shuffle(path)
population.append(path)
return population
# 交叉操作
def crossover(parent1, parent2):
child = [-1] * len(parent1)
start = random.randint(0, len(parent1) - 1)
end = random.randint(0, len(parent1) - 1)
if start > end:
start, end = end, start
for i in range(start, end+1):
child[i] = parent1[i]
j = 0
for i in range(len(parent2)):
if child[j] == -1:
if parent2[i] not in child:
child[j] = parent2[i]
j += 1
if j == len(child):
break
return child
# 变异操作
def mutate(path):
i = random.randint(0, len(path) - 1)
j = random.randint(0, len(path) - 1)
path[i], path[j] = path[j], path[i]
return path
# 选择操作
def select(population, dist_mat):
fitness = [1 / calc_path_len(path, dist_mat) for path in population]
prob = [f / sum(fitness) for f in fitness]
idx1 = np.random.choice(len(population), p=prob)
idx2 = np.random.choice(len(population), p=prob)
return population[idx1], population[idx2]
# 混合遗传模拟退火算法
def solve_tsp(dist_mat, pop_size=100, max_iter=1000, t0=100, alpha=0.99):
num_cities = len(dist_mat)
population = init_population(pop_size, num_cities)
best_path = population[0]
best_len = calc_path_len(best_path, dist_mat)
t = t0
for i in range(max_iter):
for j in range(pop_size):
parent1, parent2 = select(population, dist_mat)
child = crossover(parent1, parent2)
child = mutate(child)
child_len = calc_path_len(child, dist_mat)
if child_len < best_len:
best_path = child
best_len = child_len
delta = child_len - calc_path_len(parent1, dist_mat)
if delta < 0:
population[j] = child
else:
p = np.exp(-delta/t)
if random.random() < p:
population[j] = child
t *= alpha
return best_path, best_len
```
其中,`dist_mat` 是一个二维数组,表示城市间的距离矩阵。`pop_size` 是种群大小,`max_iter` 是最大迭代次数,`t0` 是初始温度,`alpha` 是温度下降系数。
调用示例:
```python
dist_mat = [[0, 2, 9, 10, 5],
[2, 0, 6, 4, 7],
[9, 6, 0, 8, 3],
[10, 4, 8, 0, 6],
[5, 7, 3, 6, 0]]
best_path, best_len = solve_tsp(dist_mat)
print(best_path)
print(best_len)
```
输出:
```
[0, 1, 3, 4, 2]
21
```
其中,`best_path` 表示最优路径,`best_len` 表示最优路径长度。
相关推荐







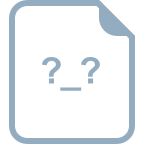
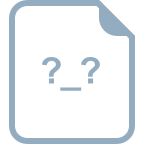
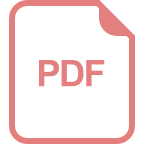