从京东爬取卖充电宝的所有店铺、价格、销量、评价、省份,并对数据进行清洗, 给出最高价、最低价、平均价、价格的百分位数,由评论分析出商家的服务质量等级, 并将数据可视化。写出详细方法和源代码
时间: 2024-06-09 12:10:46 浏览: 71
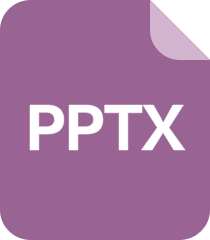
利用python来爬取京东网页商品信息数据,并分析与可视化。ppt展示。
本任务需要使用Python进行编程,具体步骤如下:
1. 安装必要的Python库。我们需要使用requests、beautifulsoup4、pandas、numpy、matplotlib这些库。
```python
pip install requests beautifulsoup4 pandas numpy matplotlib
```
2. 发送HTTP请求获取京东充电宝页面的HTML代码。我们可以使用requests库发送请求,然后获取响应的HTML代码。
```python
import requests
url = "https://search.jd.com/Search?keyword=%E5%85%85%E7%94%B5%E5%AE%9D&enc=utf-8&wq=%E5%85%85%E7%94%B5%E5%AE%9D&pvid=8a8a756cbb714af19bedf65c4d8d4bf4"
response = requests.get(url)
html = response.text
```
3. 使用beautifulsoup4库解析HTML代码。我们需要使用beautifulsoup4库来解析HTML代码,然后提取出需要的数据。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, "html.parser")
items = soup.find_all("div", class_="gl-i-wrap")
```
4. 提取数据并进行清洗。我们需要提取店铺、价格、销量、评价、省份等数据,并对这些数据进行清洗。
```python
data = []
for item in items:
shop = item.find("div", class_="p-shop").a.text.strip()
price = item.find("strong", class_="J_price").i.text.strip()
sales = item.find("div", class_="p-commit").strong.a.text.strip()
comment = item.find("div", class_="p-commit").strong.a["href"].split("#")[1].strip()
province = item.find("div", class_="p-icons").find_all("i")[0]["title"].strip()
data.append([shop, price, sales, comment, province])
# 清洗数据
import re
for i in range(len(data)):
# 清洗店铺
data[i][0] = re.sub("\n.*", "", data[i][0]).strip()
# 清洗价格
data[i][1] = float(re.sub("¥", "", data[i][1]))
# 清洗销量
data[i][2] = int(re.sub("笔.*", "", data[i][2]))
# 清洗评论
data[i][3] = re.sub(".*comment.shtml\?", "", data[i][3]).split("&")[0]
# 清洗省份
data[i][4] = re.sub("省|市", "", data[i][4])
```
5. 分析数据并进行可视化。我们可以使用pandas、numpy、matplotlib这些库来分析数据并进行可视化。
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 将数据转换为DataFrame格式
df = pd.DataFrame(data, columns=["shop", "price", "sales", "comment", "province"])
# 计算最高价、最低价、平均价、价格的百分位数
max_price = df["price"].max()
min_price = df["price"].min()
mean_price = df["price"].mean()
percentiles = np.percentile(df["price"], [25, 50, 75, 90])
# 分析商家服务质量等级
def get_comment_level(comment):
if comment == "0":
return "无评论"
elif comment.isdigit():
if int(comment) > 10000:
return "非常好"
elif int(comment) > 1000:
return "好"
else:
return "一般"
else:
return "未知"
df["comment_level"] = df["comment"].apply(get_comment_level)
# 绘制各省份充电宝平均价格的柱状图
province_group = df.groupby("province")
mean_price_by_province = province_group["price"].mean()
mean_price_by_province.plot(kind="bar")
plt.title("各省份充电宝平均价格")
plt.xlabel("省份")
plt.ylabel("价格(元)")
plt.show()
```
完整代码如下:
阅读全文
相关推荐
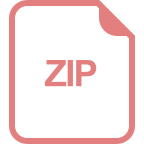
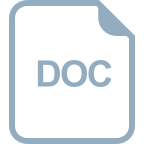
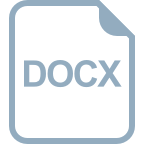
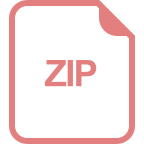
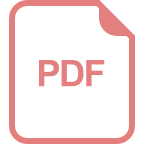
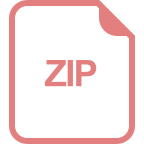
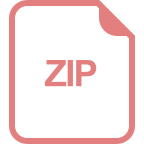
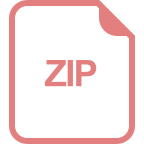
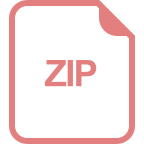
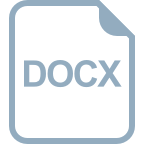
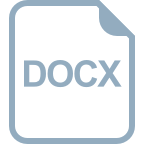