在vue3 setup 中如何使用TypeScript优化自己的代码
时间: 2024-06-04 13:11:35 浏览: 102
你好,关于在 Vue3 setup 中如何使用 TypeScript 进行优化,下面是一些建议:
1.首先,在使用 Vue3 setup 的过程中,可以在单文件组件的 script 标签中添加 lang=”ts” 属性,来启用 TypeScript,这样代码中就可以使用 TypeScript 的语法进行编写。
2.其次,可以通过声明式的方式来给 props、data、computed 等变量添加类型,这样能够有效地减少因变量类型错误造成的 bug。
3.还可以结合 Vue Composition API,使用 ref、reactive 等函数来处理组件内部的数据,更好地管理组件状态,并且可以借助 TypeScript 的类型定义来提高代码可读性和可维护性。
4.最后,可以使用 Vue3 提供的 <script setup> 语法糖,它可以让我们更加方便地使用 TypeScript,同时也可以在编译过程中帮助我们进行类型推断和错误检查。
希望以上建议能够帮助你优化自己的代码。如果还有其他问题,可以继续进行提问。
相关问题
在vue3中使用typescript
Vue 3 在设计时就考虑了 TypeScript 的支持,因此使用 TypeScript 开发 Vue 3 应用非常方便。下面是使用 TypeScript 开发 Vue 3 应用的步骤:
1. 创建一个 TypeScript 项目
首先需要创建一个 TypeScript 项目,可以使用 Vue CLI 创建一个 TypeScript 项目:
```
vue create my-project
```
在创建项目时选择 TypeScript:
```
? Please pick a preset: Manually select features
? Check the features needed for your project: Choose Vue version, Babel, TypeScript, Linter
? Choose a version of Vue.js that you want to start the project with 3.x
? Use class-style component syntax? No
? Use Babel alongside TypeScript for auto-detected polyfills? Yes
? Use history mode for router? (Requires proper server setup for index fallback in production) No
? Pick a linter / formatter config: Prettier
? Pick additional lint features: Lint on save
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated config files
```
2. 安装 Vue 3
因为 Vue 3 目前还处于 beta 版本,所以需要安装 `vue@next`。
```
npm install vue@next --save
```
3. 编写组件
在 TypeScript 中编写 Vue 组件需要使用类的形式,例如:
```typescript
import { defineComponent } from 'vue';
export default defineComponent({
name: 'HelloWorld',
props: {
msg: String,
},
setup(props) {
return {
msg: props.msg,
};
},
});
```
4. 配置 TypeScript
在 Vue 3 中使用 TypeScript 需要做一些配置,如在 `tsconfig.json` 中添加 `Vue` 的声明文件:
```json
{
"compilerOptions": {
"target": "esnext",
"module": "esnext",
"strict": true,
"jsx": "preserve",
"importHelpers": true,
"moduleResolution": "node",
"experimentalDecorators": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"allowSyntheticDefaultImports": true,
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true
},
"include": ["src", "tests", "typings", "shims-vue.d.ts"],
"exclude": ["node_modules"],
"files": ["./node_modules/vue/types/vue.d.ts"]
}
```
还需要在 `shims-vue.d.ts` 中声明 Vue 类型:
```typescript
declare module '*.vue' {
import { defineComponent } from 'vue';
const component: ReturnType<typeof defineComponent>;
export default component;
}
declare module 'vue' {
export interface ComponentCustomProperties {
$store: Store<State>;
}
}
```
5. 使用组件
在 Vue 3 中使用 TypeScript 编写的组件和使用 JavaScript 编写的组件是一样的,只需要像平常一样引入组件即可:
```vue
<template>
<HelloWorld msg="Welcome to Your Vue.js + TypeScript App"/>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import HelloWorld from '@/components/HelloWorld.vue';
export default defineComponent({
components: {
HelloWorld,
},
});
</script>
```
以上就是在 Vue 3 中使用 TypeScript 的步骤。需要注意的是,因为 Vue 3 目前还处于 beta 版本,所以在使用时可能会遇到一些问题。
typescript vue3 setup 防抖
在 TypeScript 和 Vue 3 中实现防抖的方式有多种方法,以下是其中一种常见的实现方式:
首先,你需要安装 `lodash` 库,因为我们将使用它提供的 `debounce` 方法来实现防抖功能。
```shell
npm install lodash
```
然后,在你的 Vue 3 组件中,你可以使用 `setup` 函数来定义防抖逻辑。在 `setup` 函数中,你可以使用 `ref` 创建响应式的变量,以及 `watch` 监听变量的变化。
```typescript
import { ref, watch } from 'vue';
import { debounce } from 'lodash';
export default {
setup() {
const inputValue = ref('');
// 创建一个防抖的函数
const debouncedSearch = debounce((value: string) => {
// 在这里执行需要进行防抖的操作
console.log('搜索:', value);
}, 500); // 设置防抖延迟时间为 500 毫秒
// 监听 inputValue 变化,并在变化时执行防抖函数
watch(inputValue, (value: string) => {
debouncedSearch(value);
});
return {
inputValue,
};
},
};
```
在上面的代码中,我们创建了一个名为 `inputValue` 的响应式变量,用于保存输入框的值。然后,我们使用 `debounce` 方法创建了一个名为 `debouncedSearch` 的防抖函数,它会在输入框的值变化后执行。最后,我们使用 `watch` 监听 `inputValue` 变化,并在变化时执行防抖函数。
这样,当用户在输入框中输入时,防抖函数将会延迟执行,只有在用户停止输入一段时间后才会真正执行搜索操作。这可以避免频繁的搜索请求。
阅读全文
相关推荐
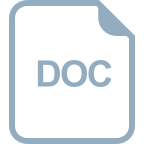
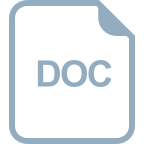
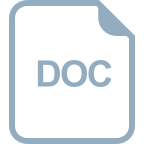
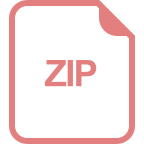







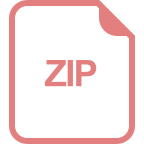
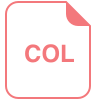



