java众微我上传了一个zip压缩包,@RequestParam("file") MultipartFile file,我改如何解压并返回所有的文件
时间: 2024-09-13 18:09:45 浏览: 41
在Java中,你可以使用Spring框架提供的MultipartFile来处理上传的zip压缩包。首先,你需要将上传的zip文件保存到服务器的临时目录,然后使用ZipInputStream或类似的库来读取并解压。以下是一个简单的步骤:
1. 检查文件是否上传成功:
```java
if (file.isEmpty()) {
throw new IllegalArgumentException("File is empty");
}
String tempDirectory = "/path/to/your/temp/directory"; // 服务器临时目录
String zipFileName = Path.of(tempDirectory, file.getOriginalFilename()).toString();
try (OutputStream outputStream = new FileOutputStream(zipFileName)) {
file.transferTo(outputStream);
}
```
2. 创建一个ZipInputStream来解压文件:
```java
ZipInputStream zis = new ZipInputStream(new FileInputStream(zipFileName));
ZipEntry entry;
while ((entry = zis.getNextEntry()) != null) {
String extractedFilePath = tempDirectory + File.separator + entry.getName(); // 解压后的路径
File dir = new File(extractedFilePath).getParentFile();
if (!dir.exists()) {
dir.mkdirs();
}
try (BufferedInputStream in = new BufferedInputStream(zis);
OutputStream out = new FileOutputStream(extractedFilePath)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
} finally {
zis.closeEntry();
}
}
zis.close();
```
3. 返回解压后的文件列表:
```java
List<File> filesInArchive = new ArrayList<>();
Files.list(Paths.get(tempDirectory)).forEach(file -> {
if (file.isDirectory() || !file.getName().startsWith("file_")) continue; // 过滤无关文件
filesInArchive.add(file.toFile());
});
// 返回文件列表
return filesInArchive;
```
阅读全文
相关推荐
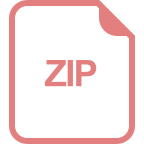
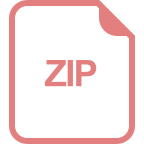
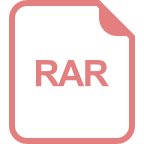




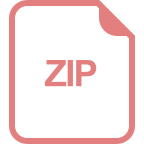
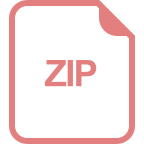
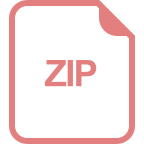
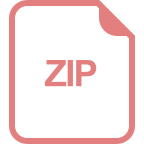






