编写一个c程序,满足以下要求: 用系统调用列出所有的信号;(kill –l) 用系统调用signal()让父进程捕捉键盘上来的中断信号(Ctrl-C); 当捕捉到中断信号后,父进程用系统调用kill()向两个子进程发出信号,子进程捕捉到信号后分别输出下列信息后终止 Child Process 1 is Killed by Parent! Child Process 2 is Killed by Parent! 父进程等待两个子进程终止后,输出如下的信息后终止: Parent Process is Killed!
时间: 2024-02-12 18:04:57 浏览: 31
下面是一个满足要求的C程序:
```c
#include <stdio.h>
#include <signal.h>
#include <unistd.h>
#include <stdlib.h>
void child_handler(int signum) {
if (signum == SIGUSR1) {
printf("Child Process 1 is Killed by Parent!\n");
exit(0);
} else if (signum == SIGUSR2) {
printf("Child Process 2 is Killed by Parent!\n");
exit(0);
}
}
int main() {
// 列出所有信号
system("kill -l");
// 注册信号处理函数
signal(SIGINT, SIG_IGN); // 忽略中断信号,交由父进程处理
signal(SIGUSR1, child_handler); // 子进程1捕捉到该信号后结束
signal(SIGUSR2, child_handler); // 子进程2捕捉到该信号后结束
// 创建子进程1
pid_t pid1 = fork();
if (pid1 == 0) {
// 子进程1
printf("Child Process 1 is Running!\n");
while (1) {
// 子进程1不断循环
}
} else if (pid1 > 0) {
// 父进程
printf("Parent Process is Running! PID=%d\n", getpid());
// 创建子进程2
pid_t pid2 = fork();
if (pid2 == 0) {
// 子进程2
printf("Child Process 2 is Running!\n");
while (1) {
// 子进程2不断循环
}
} else if (pid2 > 0) {
// 父进程
// 等待子进程1和子进程2结束
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
// 输出结束信息
printf("Parent Process is Killed!\n");
}
}
return 0;
}
```
该程序首先使用 `system("kill -l")` 执行 `kill -l` 命令,列出所有信号。然后父进程注册了处理中断信号的函数 `child_handler`,并通过 `signal()` 系统调用将中断信号 `SIGINT` 交由父进程处理。接着,父进程创建了两个子进程,并分别注册了处理信号的函数 `child_handler`,用于捕捉由父进程发出的 `SIGUSR1` 和 `SIGUSR2` 信号。子进程1和子进程2在循环中不断运行,直到接收到信号后输出相应的信息并结束。最后,父进程等待两个子进程结束后输出结束信息并退出。
相关推荐
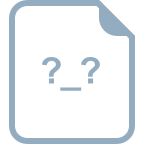
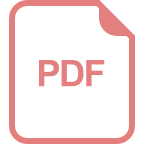
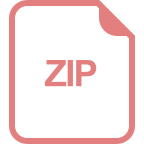
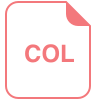
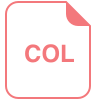
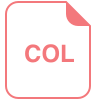
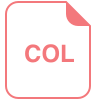
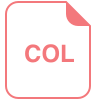








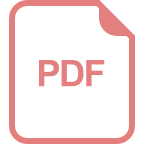
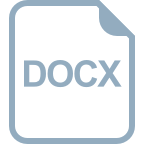