使用c++和汇编语言混合编程,devc++中执行,内嵌调用 程序例子5个
时间: 2024-01-22 10:18:46 浏览: 109
以下是使用C++和汇编语言混合编程的5个程序例子,这些例子可以在Dev-C++中执行,并且使用了内嵌调用。
1. 计算两个整数的和
```c++
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 20, sum;
__asm {
mov eax, a
add eax, b
mov sum, eax
}
cout << "The sum of " << a << " and " << b << " is " << sum << endl;
return 0;
}
```
2. 将一段字符串反转
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[] = "Hello, world!";
int len = strlen(str);
__asm {
mov esi, offset str
mov edi, esi
add edi, len
dec edi
loop1:
cmp esi, edi
jg done
mov al, [esi]
mov bl, [edi]
mov [esi], bl
mov [edi], al
inc esi
dec edi
jmp loop1
done:
}
cout << "Reversed string: " << str << endl;
return 0;
}
```
3. 计算阶乘
```c++
#include <iostream>
using namespace std;
int main() {
int n = 5, fact = 1;
__asm {
mov ecx, n
mov eax, 1
loop1:
cmp ecx, 0
je done
mul ecx
dec ecx
jmp loop1
done:
mov fact, eax
}
cout << "Factorial of " << n << " is " << fact << endl;
return 0;
}
```
4. 判断一个数是否为素数
```c++
#include <iostream>
using namespace std;
int main() {
int n = 17, flag = 0;
__asm {
mov ecx, n
mov ebx, 2
loop1:
cmp ebx, ecx
jge done
mov edx, 0
div ebx
cmp edx, 0
je composite
inc ebx
jmp loop1
composite:
mov flag, 1
jmp done
done:
}
if (flag)
cout << n << " is not a prime number" << endl;
else
cout << n << " is a prime number" << endl;
return 0;
}
```
5. 将一个数组中的元素翻倍
```c++
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
__asm {
mov ecx, n
mov esi, offset arr
loop1:
cmp ecx, 0
je done
mov eax, [esi]
add eax, eax
mov [esi], eax
add esi, 4
dec ecx
jmp loop1
done:
}
cout << "Doubled array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
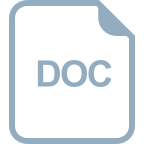
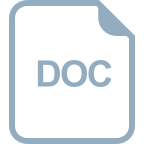
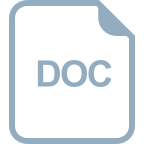




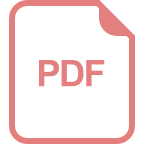
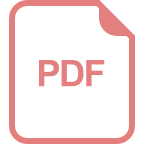
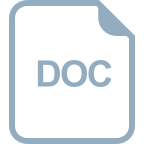
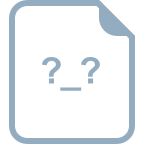
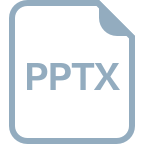
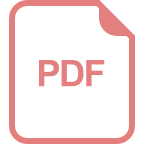
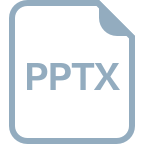
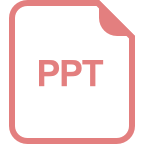
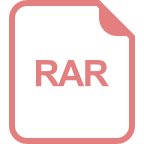
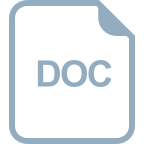
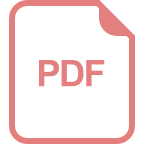
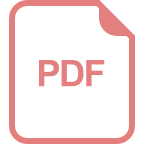