设计一个计算机程序,能够实现简单的员工管理功能。员工信息有随机数生成器生成,员工信息包括:编号、姓名、性别、出生年月、学历、职务、电话、住址
时间: 2023-07-04 07:11:16 浏览: 214
好的,我可以帮您设计一个员工管理系统的计算机程序。
首先,我们需要定义员工的信息包括编号、姓名、性别、出生年月、学历、职务、电话、住址。为了方便起见,我们可以使用结构体来存储员工的信息。
接下来,我们需要设计程序的功能。根据您的要求,我们需要实现以下功能:
1. 添加员工信息:程序可以通过随机数生成器生成员工信息,并将其添加到员工信息库中。
2. 删除员工信息:程序可以根据员工编号或姓名删除指定员工的信息。
3. 修改员工信息:程序可以根据员工编号或姓名修改指定员工的信息。
4. 查询员工信息:程序可以根据员工编号或姓名查询指定员工的信息。
5. 显示所有员工信息:程序可以显示当前员工信息库中所有员工的信息。
我们可以使用 C++ 编程语言来实现这个程序,以下是示例代码:
```cpp
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
struct Employee {
string number;
string name;
string gender;
string birth;
string education;
string job;
string phone;
string address;
};
// 添加员工信息
void addEmployee(Employee employees[], int& count) {
// 生成随机数
srand((unsigned)time(nullptr));
employees[count].number = to_string(rand()%10000+1000);
employees[count].name = "Employee " + to_string(count+1);
employees[count].gender = (rand()%2 == 0) ? "Male" : "Female";
employees[count].birth = to_string(rand()%21+1980) + "/" + to_string(rand()%12+1) + "/" + to_string(rand()%28+1);
employees[count].education = "Education " + to_string(rand()%5+1);
employees[count].job = "Job " + to_string(rand()%5+1);
employees[count].phone = "138" + to_string(rand()%90000000+10000000);
employees[count].address = "Address " + to_string(rand()%10+1);
count++;
cout << "员工信息添加成功!" << endl;
}
// 删除员工信息
void deleteEmployee(Employee employees[], int& count) {
string numberOrName;
cout << "请输入要删除的员工编号或姓名:";
cin >> numberOrName;
bool flag = false;
for (int i = 0; i < count; i++) {
if (employees[i].number == numberOrName || employees[i].name == numberOrName) {
for (int j = i; j < count-1; j++) {
employees[j] = employees[j+1];
}
count--;
flag = true;
cout << "员工信息删除成功!" << endl;
break;
}
}
if (!flag) {
cout << "未找到对应员工信息!" << endl;
}
}
// 修改员工信息
void modifyEmployee(Employee employees[], int count) {
string numberOrName;
cout << "请输入要修改的员工编号或姓名:";
cin >> numberOrName;
bool flag = false;
for (int i = 0; i < count; i++) {
if (employees[i].number == numberOrName || employees[i].name == numberOrName) {
cout << "请输入员工编号:";
cin >> employees[i].number;
cout << "请输入员工姓名:";
cin >> employees[i].name;
cout << "请输入员工性别:";
cin >> employees[i].gender;
cout << "请输入员工出生日期(yyyy/mm/dd):";
cin >> employees[i].birth;
cout << "请输入员工学历:";
cin >> employees[i].education;
cout << "请输入员工职务:";
cin >> employees[i].job;
cout << "请输入员工电话:";
cin >> employees[i].phone;
cout << "请输入员工地址:";
cin >> employees[i].address;
flag = true;
cout << "员工信息修改成功!" << endl;
break;
}
}
if (!flag) {
cout << "未找到对应员工信息!" << endl;
}
}
// 查询员工信息
void queryEmployee(Employee employees[], int count) {
string numberOrName;
cout << "请输入要查询的员工编号或姓名:";
cin >> numberOrName;
bool flag = false;
for (int i = 0; i < count; i++) {
if (employees[i].number == numberOrName || employees[i].name == numberOrName) {
cout << "编号:" << employees[i].number << endl;
cout << "姓名:" << employees[i].name << endl;
cout << "性别:" << employees[i].gender << endl;
cout << "出生日期:" << employees[i].birth << endl;
cout << "学历:" << employees[i].education << endl;
cout << "职务:" << employees[i].job << endl;
cout << "电话:" << employees[i].phone << endl;
cout << "地址:" << employees[i].address << endl;
flag = true;
break;
}
}
if (!flag) {
cout << "未找到对应员工信息!" << endl;
}
}
// 显示所有员工信息
void displayEmployees(Employee employees[], int count) {
for (int i = 0; i < count; i++) {
cout << "编号:" << employees[i].number << endl;
cout << "姓名:" << employees[i].name << endl;
cout << "性别:" << employees[i].gender << endl;
cout << "出生日期:" << employees[i].birth << endl;
cout << "学历:" << employees[i].education << endl;
cout << "职务:" << employees[i].job << endl;
cout << "电话:" << employees[i].phone << endl;
cout << "地址:" << employees[i].address << endl << endl;
}
}
int main() {
int choice;
int count = 0;
Employee employees[100];
while (true) {
cout << "请选择要进行的操作:" << endl;
cout << "1. 添加员工信息" << endl;
cout << "2. 删除员工信息" << endl;
cout << "3. 修改员工信息" << endl;
cout << "4. 查询员工信息" << endl;
cout << "5. 显示所有员工信息" << endl;
cout << "6. 退出程序" << endl;
cin >> choice;
switch (choice) {
case 1:
addEmployee(employees, count);
break;
case 2:
deleteEmployee(employees, count);
break;
case 3:
modifyEmployee(employees, count);
break;
case 4:
queryEmployee(employees, count);
break;
case 5:
displayEmployees(employees, count);
break;
case 6:
return 0;
default:
cout << "无效操作!" << endl;
break;
}
}
return 0;
}
```
这个程序可以实现您所需要的员工管理功能,您可以根据实际需求进行修改和完善。
阅读全文
相关推荐
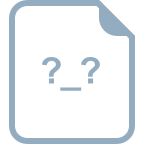

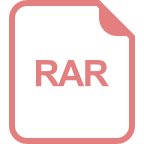








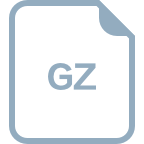

