用quartus设计一个简单可重构阵列计算模块、操作模块、的vhdl代码,
时间: 2023-11-13 09:02:54 浏览: 35
好的,下面是可重构阵列的计算模块和操作模块的简单VHDL代码示例:
1. 计算模块
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity ComputeUnit is
generic (
DATA_WIDTH : integer := 32 -- 数据位宽
);
port (
clk : in std_logic; -- 时钟
din1 : in std_logic_vector(DATA_WIDTH - 1 downto 0); -- 输入数据1
din2 : in std_logic_vector(DATA_WIDTH - 1 downto 0); -- 输入数据2
op_sel : in std_logic_vector(1 downto 0); -- 运算选择
dout : out std_logic_vector(DATA_WIDTH - 1 downto 0) -- 输出数据
);
end ComputeUnit;
architecture Behavioral of ComputeUnit is
begin
process (clk)
begin
if rising_edge(clk) then
case op_sel is
when "00" => -- 加法
dout <= std_logic_vector(unsigned(din1) + unsigned(din2));
when "01" => -- 减法
dout <= std_logic_vector(unsigned(din1) - unsigned(din2));
when "10" => -- 乘法
dout <= std_logic_vector(unsigned(din1) * unsigned(din2));
when others => -- 未定义
dout <= (others => 'X');
end case;
end if;
end process;
end Behavioral;
```
2. 操作模块
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity ControlUnit is
generic (
ADDR_WIDTH : integer := 8 -- 地址位宽
);
port (
clk : in std_logic; -- 时钟
din : in std_logic_vector(3 downto 0); -- 输入数据
addr : in std_logic_vector(ADDR_WIDTH - 1 downto 0); -- 操作地址
wr_en : in std_logic; -- 写使能
dout : out std_logic_vector(3 downto 0) -- 输出数据
);
end ControlUnit;
architecture Behavioral of ControlUnit is
type reg_type is array (0 to 2**ADDR_WIDTH-1) of std_logic_vector(3 downto 0);
signal reg : reg_type := (others => (others => '0')); -- 初始化为全0
begin
process (clk)
begin
if rising_edge(clk) then
if wr_en = '1' then
-- 写操作
reg(to_integer(unsigned(addr))) <= din;
end if;
-- 读操作
dout <= reg(to_integer(unsigned(addr)));
end if;
end process;
end Behavioral;
```
其中,计算模块用于执行加法、减法和乘法等基本运算;操作模块用于读写控制寄存器,控制计算模块的运行。在实际应用中,还需要根据具体的计算任务来设计和实现可重构阵列的逻辑和控制模块。
相关推荐
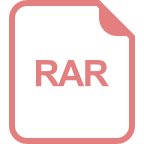
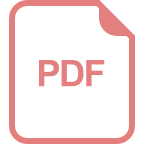














