堆栈迷宫问题C语言代码
时间: 2024-10-18 17:13:28 浏览: 32
在C语言中,使用堆栈来解决迷宫问题通常结合了深度优先搜索(DFS)策略。以下是一个简化的示例代码,假设我们有一个二维字符数组`maze`表示迷宫,'.' 表示空地,'#' 表示墙壁,S 和 E 分别代表起始点和结束点:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 5
#define COLS 5
typedef struct {
int row, col;
} Cell;
typedef struct {
Cell position;
bool visited;
} StackElement;
typedef struct {
StackElement elements[ROWS * COLS];
int top;
} Stack;
// 初始化堆栈
Stack stack_init() {
Stack s;
s.top = -1;
return s;
}
// 判断当前位置是否有效
bool is_valid(int row, int col, char maze[ROWS][COLS]) {
return row >= 0 && row < ROWS && col >= 0 && col < COLS && maze[row][col] == '.';
}
// 检查目标到达
bool is_goal(Cell cell, Cell goal) {
return cell.row == goal.row && cell.col == goal.col;
}
// 添加元素到堆栈
void push(Stack* s, StackElement* element) {
element->visited = true;
s->elements[++s->top] = *element;
}
// 弹出堆栈顶部元素
StackElement* pop(Stack* s) {
return &s->elements[s->top--];
}
// 主函数:使用DFS
void solve_maze(char maze[ROWS][COLS], Cell start, Cell end) {
Stack s = stack_init();
StackElement start_element = {start, false};
push(&s, &start_element);
while (s.top != -1) {
StackElement current = pop(&s);
if (is_goal(current.position, end)) {
trace_path(s, current);
break;
}
for (int i = -1; j <= 1; j++) {
Cell neighbor = {current.position.row + i, current.position.col + j};
if (is_valid(neighbor.row, neighbor.col, maze) && !current.visited) {
StackElement next_element = {neighbor, false};
push(&s, &next_element);
}
}
}
}
}
// 打印路径
void trace_path(Stack s, StackElement current) {
printf("(%d,%d)", current.position.row, current.position.col);
while (current.position.row != start.position.row || current.position.col != start.position.col) {
current = pop(&s);
printf(" -> (%d,%d)", current.position.row, current.position.col);
}
printf("\n");
}
int main() {
// 初始化迷宫...
char maze[ROWS][COLS] = {
{'#', '.', '#', '.', '.'},
{'.', 'S', '.', '#', '.'},
{'.', '.', '.', '#', '.'},
{'.', '.', '#', '.', 'E'},
{'#', '.', '#', '.', '.'}
};
Cell start = {1, 1}, end = {4, 4};
solve_maze(maze, start, end);
return 0;
}
```
请注意,这只是一个基本示例,实际应用中可能需要根据具体需求进行调整。同时,此代码并未处理错误边界和边界情况,实际编写时应增加相应的检查。
阅读全文
相关推荐
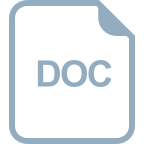
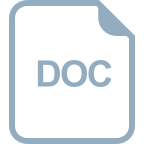
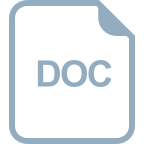

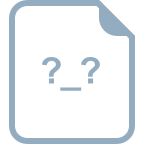
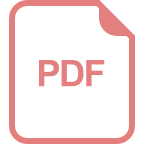
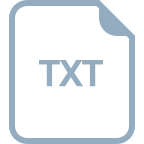
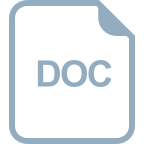
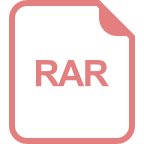
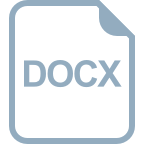
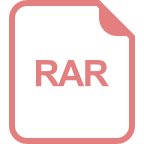
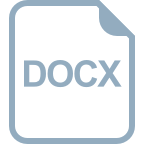
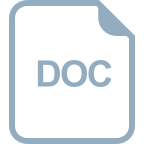
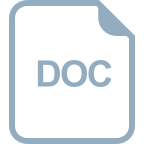


