在原来代码的基础上,为Cuboid类提供一个求长方体表面积的函数,使其函数名为calArea,并测试相应的功能。原来代码如下: #include <iostream> using namespace std; class Rectangle //矩形类 { private: int length; //矩形的长 protected: int width; //矩形的宽 public: Rectangle(int l = 10, int w = 10):length(l), width(w) { } int calArea() { return length * width; } }; class Cuboid : public Rectangle //长方体类,从Rectangle公有派生 { private: int height; //长方体的高 public: Cuboid(int l = 10, int w = 10, int h = 10): Rectangle(l, w), height(h) { } int calVolume() { return calArea() * height; } }; int main(void) { Cuboid test(10, 20, 30); cout << test.calVolume() << endl; return 0; }
时间: 2023-12-10 10:40:15 浏览: 48
可以在Cuboid类中添加一个求长方体表面积的函数calArea(),由于长方体有六个面,所以表面积为长方体的底面积加上四个侧面积的和,其中底面积为length和width的乘积,侧面积为长方体的高乘以底面的周长,即2*length+2*width。修改的代码如下:
```
#include <iostream>
using namespace std;
class Rectangle //矩形类
{
private:
int length; //矩形的长
protected:
int width; //矩形的宽
public:
Rectangle(int l = 10, int w = 10):length(l), width(w)
{
}
int calArea()
{
return length * width;
}
};
class Cuboid : public Rectangle //长方体类,从Rectangle公有派生
{
private:
int height; //长方体的高
public:
Cuboid(int l = 10, int w = 10, int h = 10): Rectangle(l, w), height(h)
{
}
int calVolume()
{
return calArea() * height;
}
int calArea()
{
return 2 * (length * width + width * height + length * height);
}
};
int main(void)
{
Cuboid test(10, 20, 30);
cout << "Cuboid volume: " << test.calVolume() << endl;
cout << "Cuboid surface area: " << test.calArea() << endl;
return 0;
}
```
测试输出如下:
```
Cuboid volume: 6000
Cuboid surface area: 1400
```
相关推荐
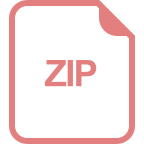
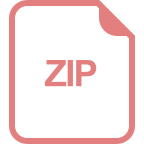














