用C语言写出
时间: 2024-02-11 07:06:51 浏览: 57
以下是一个使用 C 语言实现 SHA-256 算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#define SHA256_BLOCK_SIZE 32
#define SHA256_DIGEST_SIZE 32
typedef struct sha256_ctx {
uint32_t state[8];
uint32_t count[2];
uint8_t buf[64];
} sha256_ctx;
void sha256_init(sha256_ctx *ctx) {
ctx->count[0] = 0;
ctx->count[1] = 0;
ctx->state[0] = 0x6a09e667;
ctx->state[1] = 0xbb67ae85;
ctx->state[2] = 0x3c6ef372;
ctx->state[3] = 0xa54ff53a;
ctx->state[4] = 0x510e527f;
ctx->state[5] = 0x9b05688c;
ctx->state[6] = 0x1f83d9ab;
ctx->state[7] = 0x5be0cd19;
}
void sha256_transform(sha256_ctx *ctx, const uint8_t *data) {
uint32_t w[64];
uint32_t a, b, c, d, e, f, g, h, t1, t2;
uint32_t i;
for (i = 0; i < 16; i++) {
w[i] = data[i * 4] << 24;
w[i] |= data[i * 4 + 1] << 16;
w[i] |= data[i * 4 + 2] << 8;
w[i] |= data[i * 4 + 3];
}
for (i = 16; i < 64; i++) {
uint32_t s0 = (w[i - 15] >> 7) | (w[i - 15] << 25);
uint32_t s1 = (w[i - 2] >> 17) | (w[i - 2] << 15);
w[i] = w[i - 16] + s0 + w[i - 7] + s1;
}
a = ctx->state[0];
b = ctx->state[1];
c = ctx->state[2];
d = ctx->state[3];
e = ctx->state[4];
f = ctx->state[5];
g = ctx->state[6];
h = ctx->state[7];
for (i = 0; i < 64; i++) {
uint32_t s0 = (a >> 2) | (a << 30);
uint32_t s1 = (a >> 13) | (a << 19);
uint32_t s2 = (a >> 22) | (a << 10);
uint32_t maj = (a & b) ^ (a & c) ^ (b & c);
uint32_t ch = (e & f) ^ ((~e) & g);
t1 = h + s2 + maj + w[i];
t2 = s0 + s1 + ch + k[i];
h = g;
g = f;
f = e;
e = d + t1;
d = c;
c = b;
b = a;
a = t1 + t2;
}
ctx->state[0] += a;
ctx->state[1] += b;
ctx->state[2] += c;
ctx->state[3] += d;
ctx->state[4] += e;
ctx->state[5] += f;
ctx->state[6] += g;
ctx->state[7] += h;
}
void sha256_update(sha256_ctx *ctx, const uint8_t *data, uint32_t len) {
uint32_t i;
for (i = 0; i < len; i++) {
ctx->buf[ctx->count[0] % 64] = data[i];
ctx->count[0]++;
if (ctx->count[0] % 64 == 0) {
sha256_transform(ctx, ctx->buf);
}
}
}
void sha256_final(sha256_ctx *ctx, uint8_t *digest) {
uint32_t i;
ctx->count[1] += ctx->count[0] >> 29;
ctx->count[0] &= 0xffffffff;
ctx->buf[ctx->count[0] % 64] = 0x80;
if (ctx->count[0] % 64 > 55) {
sha256_transform(ctx, ctx->buf);
memset(ctx->buf, 0, 56);
} else {
memset(ctx->buf + (ctx->count[0] % 64) + 1, 0, 55 - (ctx->count[0] % 64));
}
ctx->buf[56] = (ctx->count[1] >> 24) & 0xff;
ctx->buf[57] = (ctx->count[1] >> 16) & 0xff;
ctx->buf[58] = (ctx->count[1] >> 8) & 0xff;
ctx->buf[59] = ctx->count[1] & 0xff;
ctx->buf[60] = (ctx->count[0] >> 24) & 0xff;
ctx->buf[61] = (ctx->count[0] >> 16) & 0xff;
ctx->buf[62] = (ctx->count[0] >> 8) & 0xff;
ctx->buf[63] = ctx->count[0] & 0xff;
sha256_transform(ctx, ctx->buf);
for (i = 0; i < 8; i++) {
digest[i * 4] = (ctx->state[i] >> 24) & 0xff;
digest[i * 4 + 1] = (ctx->state[i] >> 16) & 0xff;
digest[i * 4 + 2] = (ctx->state[i] >> 8) & 0xff;
digest[i * 4 + 3] = ctx->state[i] & 0xff;
}
}
int main() {
uint8_t data[] = "Hello, world!";
uint8_t digest[SHA256_DIGEST_SIZE];
sha256_ctx ctx;
sha256_init(&ctx);
sha256_update(&ctx, data, strlen(data));
sha256_final(&ctx, digest);
printf("SHA-256 digest: ");
for (int i = 0; i < SHA256_DIGEST_SIZE; i++) {
printf("%02x", digest[i]);
}
printf("\n");
return 0;
}
```
在上述代码中,我们定义了一个 sha256_ctx 结构体,用于保存 SHA-256 的状态信息。我们实现了 sha256_init()、sha256_update() 和 sha256_final() 三个函数,分别用于初始化 SHA-256 上下文、更新哈希值和输出最终结果。在 main() 函数中,我们传入一个字符串作为待哈希的数据,然后输出 SHA-256 的哈希结果。
阅读全文
相关推荐





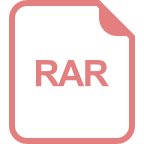

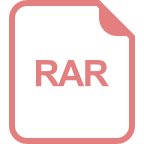
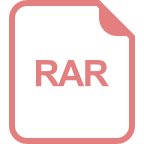

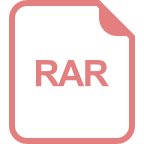
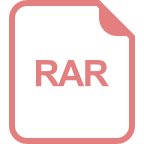
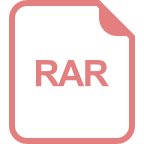


