java实现sm4
时间: 2023-10-05 07:04:19 浏览: 109
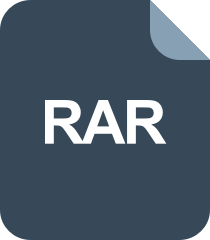
sm2、SM3、sm4国密加密算法java实现.rar
SM4是一种国密算法,Java中可以使用Bouncy Castle库来实现。
首先需要引入Bouncy Castle库,可以在Maven中添加以下依赖:
```xml
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcpkix-jdk15on</artifactId>
<version>1.66</version>
</dependency>
```
然后就可以使用以下代码实现SM4加解密:
```java
import org.bouncycastle.crypto.BlockCipher;
import org.bouncycastle.crypto.CipherParameters;
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.encoders.Hex;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
public class SM4Util {
private static final int BLOCK_SIZE = 16;
/**
* 加密
*/
public static byte[] encrypt(byte[] key, byte[] iv, byte[] data) {
SM4Engine engine = new SM4Engine();
BlockCipher cipher = new CBCBlockCipher(engine);
CipherParameters params = new ParametersWithIV(new KeyParameter(key), iv);
cipher.init(true, params);
int inputLen = data.length;
int paddingLen = BLOCK_SIZE - (inputLen % BLOCK_SIZE);
byte[] input = new byte[inputLen + paddingLen];
System.arraycopy(data, 0, input, 0, inputLen);
for (int i = 0; i < paddingLen; i++) {
input[inputLen + i] = (byte) paddingLen;
}
byte[] output = new byte[input.length];
int i = 0;
while (i < input.length) {
int len = cipher.processBlock(input, i, output, i);
i += len;
}
return output;
}
/**
* 解密
*/
public static byte[] decrypt(byte[] key, byte[] iv, byte[] data) {
SM4Engine engine = new SM4Engine();
BlockCipher cipher = new CBCBlockCipher(engine);
CipherParameters params = new ParametersWithIV(new KeyParameter(key), iv);
cipher.init(false, params);
byte[] output = new byte[data.length];
int i = 0;
while (i < data.length) {
int len = cipher.processBlock(data, i, output, i);
i += len;
}
int paddingLen = output[output.length - 1];
byte[] result = new byte[output.length - paddingLen];
System.arraycopy(output, 0, result, 0, result.length);
return result;
}
public static void main(String[] args) {
byte[] key = Hex.decode("0123456789abcdeffedcba9876543210");
byte[] iv = new byte[BLOCK_SIZE];
new SecureRandom().nextBytes(iv);
String plaintext = "Hello, world!";
byte[] ciphertext = encrypt(key, iv, plaintext.getBytes(StandardCharsets.UTF_8));
System.out.println("Ciphertext: " + Hex.toHexString(ciphertext));
byte[] decrypted = decrypt(key, iv, ciphertext);
String decryptedText = new String(decrypted, StandardCharsets.UTF_8);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
其中,加密和解密都需要传入密钥和IV,IV需要随机生成,加密时还需要进行PKCS7填充。
阅读全文
相关推荐
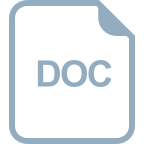
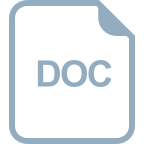
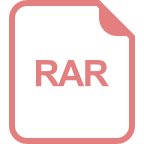











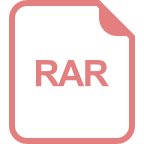
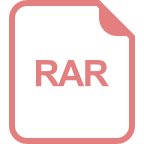