请用java实现sm4的加密过程
时间: 2024-03-21 12:42:06 浏览: 18
好的,以下是Java实现SM4加密过程的示例代码:
```java
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.paddings.PaddedBufferedBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.Arrays;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
public class SM4Encryption {
public static byte[] encrypt(byte[] key, byte[] iv, byte[] plaintext) throws Exception {
// Create the SM4 cipher engine
SM4Engine sm4engine = new SM4Engine();
// Use CBC mode with PKCS#7 padding
PaddedBufferedBlockCipher cipher = new PaddedBufferedBlockCipher(new CBCBlockCipher(sm4engine));
// Create the key and IV parameters
KeyParameter keyParam = new KeyParameter(key);
ParametersWithIV params = new ParametersWithIV(keyParam, iv);
// Initialize the cipher engine with the key and IV parameters
cipher.init(true, params);
// Encrypt the plaintext
byte[] ciphertext = new byte[cipher.getOutputSize(plaintext.length)];
int len = cipher.processBytes(plaintext, 0, plaintext.length, ciphertext, 0);
cipher.doFinal(ciphertext, len);
return ciphertext;
}
public static void main(String[] args) throws Exception {
// Generate a random 128-bit key and 128-bit IV
SecureRandom random = new SecureRandom();
byte[] key = new byte[16];
byte[] iv = new byte[16];
random.nextBytes(key);
random.nextBytes(iv);
// Encrypt a plaintext message
String plaintext = "Hello, world!";
byte[] ciphertext = encrypt(key, iv, plaintext.getBytes(StandardCharsets.UTF_8));
System.out.println("Plaintext: " + plaintext);
System.out.println("Key: " + Arrays.toString(key));
System.out.println("IV: " + Arrays.toString(iv));
System.out.println("Ciphertext: " + Arrays.toString(ciphertext));
}
}
```
在上面的示例代码中,我们使用了Bouncy Castle库中的SM4算法实现了加密。代码中首先生成一个随机的128位密钥和128位初始向量,然后使用SM4算法对明文进行加密。最后输出明文、密钥、初始向量和密文。注意,这里的加密过程是使用CBC模式和PKCS#7填充方式。
相关推荐
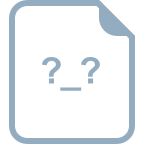
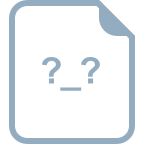
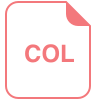
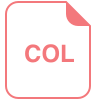
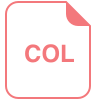
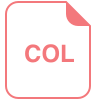










