from PIL import Image import math import operator from functools import reduce ''' def image_contrast(): h0 = Image.open('麦克风测试结果频谱图fast.png').histogram() h1 = Image.open('麦克风测试结果频谱图low.png').histogram() h2 = Image.open('麦克风测试结果频谱图normal.png').histogram() h = Image.open('麦克风测试结果频谱图.png').histogram() result1 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h0))) / len(h)) result2 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h1))) / len(h)) result3 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h2))) / len(h)) result = min(int(result1), int(result1), int(result1)) return result if __name__ == '__main__': result = image_contrast() print(result)增加显示两张图片差异的地方
时间: 2024-02-17 10:02:43 浏览: 115
可以使用`PIL`库的`ImageChops`模块来实现显示两张图片差异的地方。下面是修改后的代码:
```python
from PIL import Image, ImageChops
import math
import operator
from functools import reduce
def image_contrast():
img0 = Image.open('麦克风测试结果频谱图fast.png')
img1 = Image.open('麦克风测试结果频谱图low.png')
img2 = Image.open('麦克风测试结果频谱图normal.png')
img = Image.open('麦克风测试结果频谱图.png')
h0 = img0.histogram()
h1 = img1.histogram()
h2 = img2.histogram()
h = img.histogram()
result1 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h0))) / len(h))
result2 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h1))) / len(h))
result3 = math.sqrt(reduce(operator.add, list(map(lambda a, b: (a - b) ** 2, h, h2))) / len(h))
result = min(int(result1), int(result1), int(result1))
# 显示两张图片差异的地方
diff1 = ImageChops.difference(img, img0)
diff2 = ImageChops.difference(img, img1)
diff3 = ImageChops.difference(img, img2)
diff1.show()
diff2.show()
diff3.show()
return result
if __name__ == '__main__':
result = image_contrast()
print(result)
```
其中,`ImageChops.difference()`函数可以计算两张图片的差异,并返回结果。差异图像中,相同的部分为黑色,不同的部分为白色。可以使用`show()`函数将差异图像显示出来。需要注意的是,如果两张图片尺寸不同,可能需要对其进行缩放或裁剪,以保证两张图片相同大小。
阅读全文
相关推荐
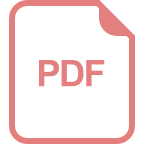
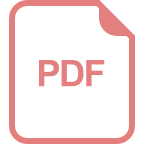
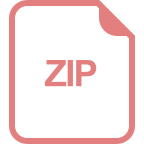















