regex_replace函数的使用
时间: 2023-08-19 20:09:53 浏览: 175
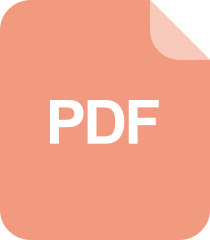
javascript 正则替换 replace(regExp, function)用法
regex_replace函数是C++11标准库中的一个函数,用于在字符串中查找并替换符合正则表达式的匹配项。它的定义如下:
```c++
template <class BidirIt, class Traits, class CharT, class ST>
basic_string<CharT, Traits, ST> regex_replace(
BidirIt first, BidirIt last,
const basic_regex<CharT, Traits>& re,
const basic_string<CharT, Traits, ST>& fmt,
regex_constants::match_flag_type flags =
regex_constants::match_default);
```
其中,`first`和`last`表示被查找和替换的字符串范围;`re`表示正则表达式;`fmt`是替换的格式字符串;`flags`表示匹配标志,默认为`regex_constants::match_default`。
下面是一个简单的示例代码,使用正则表达式将字符串中所有数字替换为`x`:
```c++
#include <iostream>
#include <regex>
int main() {
std::string str = "hello123world456";
std::regex re("\\d+");
std::string fmt("x");
std::string result = std::regex_replace(str, re, fmt);
std::cout << result << std::endl;
return 0;
}
```
输出:
```
helloxworldx
```
在这个示例中,使用`std::regex`构造了一个正则表达式,匹配所有数字`\d+`,然后用字符串`x`替换所有匹配项。最后输出替换后的字符串。
需要注意的是,`regex_replace`函数返回的是一个新的字符串,而不是原字符串的引用。如果需要直接修改原字符串,可以使用`std::regex_replace`的另一个重载函数:
```c++
template <class Traits, class CharT, class ST, class UnaryFunction>
basic_string<CharT, Traits, ST> regex_replace(
const basic_string<CharT, Traits, ST>& s,
const basic_regex<CharT, Traits>& re,
UnaryFunction f,
regex_constants::match_flag_type flags =
regex_constants::match_default);
```
其中,`f`表示替换函数,它接受一个`std::smatch`对象作为参数,返回一个替换后的字符串。这个函数可以直接修改原字符串,例如:
```c++
#include <iostream>
#include <regex>
int main() {
std::string str = "hello123world456";
std::regex re("\\d+");
std::string result = std::regex_replace(str, re, [](const std::smatch& m) {
return "x";
});
std::cout << result << std::endl;
return 0;
}
```
输出:
```
helloxworldx
```
在这个示例中,使用一个匿名Lambda函数作为替换函数,将所有匹配项替换为字符串`x`。由于是直接修改原字符串,输出结果与前面的示例相同。
阅读全文
相关推荐
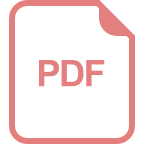
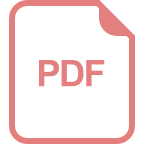
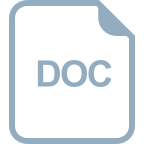







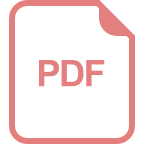
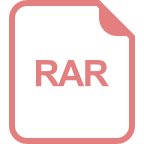
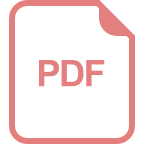
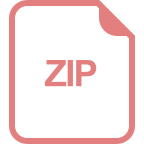


