按下列要求编写程序: 1. 定义学生信息的栈数据结构 (包括 学号 姓名 成绩) 2. 设计栈的初始化、入栈、出栈等功能函数,实现学生信息的依次入栈与出栈 3. 编写程序主函数,对各个功能函数进行调用 4. 物理存储结构不限,顺序存储或者链式存储均可
时间: 2023-05-29 16:04:31 浏览: 72
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 100
typedef struct {
char id[10];
char name[20];
int score;
} Student;
typedef struct {
Student data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void initStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack *s) {
return s->top == MAXSIZE - 1;
}
// 入栈
void push(Stack *s, Student stu) {
if (isFull(s)) {
printf("Error: Stack is full!\n");
return;
}
s->top++;
s->data[s->top] = stu;
}
// 出栈
Student pop(Stack *s) {
if (isEmpty(s)) {
printf("Error: Stack is empty!\n");
exit(0);
}
return s->data[s->top--];
}
int main() {
Stack s;
initStack(&s);
int choice;
while (1) {
printf("1. Push student information\n");
printf("2. Pop student information\n");
printf("3. Quit\n");
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
if (isFull(&s)) {
printf("Error: Stack is full!\n");
break;
}
Student stu;
printf("Please enter student id: ");
scanf("%s", stu.id);
printf("Please enter student name: ");
scanf("%s", stu.name);
printf("Please enter student score: ");
scanf("%d", &stu.score);
push(&s, stu);
printf("Push student information successfully!\n");
break;
case 2:
if (isEmpty(&s)) {
printf("Error: Stack is empty!\n");
break;
}
Student stu = pop(&s);
printf("Student information poped: id=%s, name=%s, score=%d\n", stu.id, stu.name, stu.score);
break;
case 3:
return 0;
default:
printf("Error: Invalid choice!\n");
break;
}
}
return 0;
}
阅读全文
相关推荐
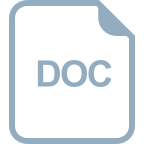
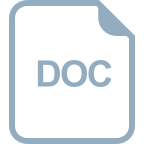
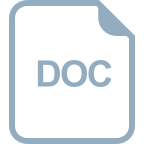


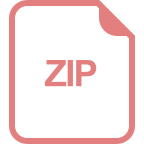
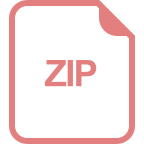
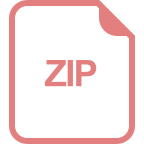

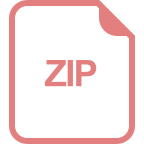
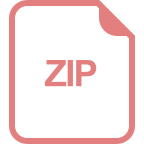
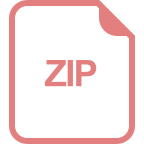
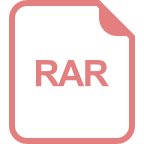
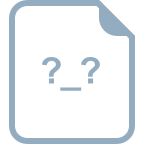
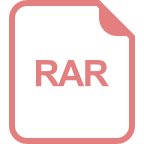
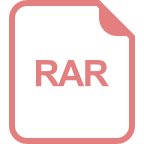
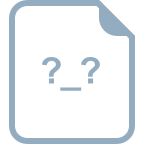
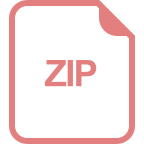
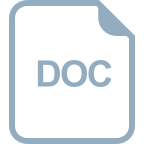