生成一段给定末端工具的位置(x, y, z)和姿态信息通过逆运动学算法来确定关节角度(u, v, w)的完整版python程序
时间: 2024-09-10 11:02:42 浏览: 62
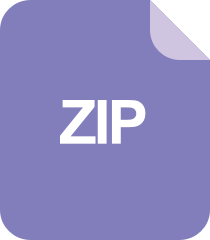
phmxm.zip_逆运动学算法
逆运动学是一个计算多关节机械臂所需关节角度的过程,以使得末端工具达到特定位置和姿态。下面是一个简单的Python程序示例,使用直接搜索方法(如线性插值)来求解。对于复杂的机器人,可能需要使用更精确的优化算法,如牛顿法或拟牛顿法。这里假设你已经有了一个预定义的DH参数表,例如KUKA LWR机器人的参数。
```python
import numpy as np
# 假设我们有DH参数
d = [0.75, 0.75, 1.5]
a = [0, 0, 0]
alpha = [np.pi/2, -np.pi/2, -np.pi/2]
theta_initial = [0, 0, 0] # 初始关节角度
def homogeneous_matrix(pose, theta):
R = np.array([[np.cos(theta[2]), -np.sin(theta[2]) * np.cos(theta[1]), np.sin(theta[2]) * np.sin(theta[1])],
[np.sin(theta[2]) * np.cos(theta[0]), np.cos(theta[2]) * np.cos(theta[0]) - np.sin(theta[1]) * np.sin(theta[0]) * np.sin(theta[2]),
np.sin(theta[0]) * np.sin(theta[1]) + np.cos(theta[1]) * np.cos(theta[2]) * np.sin(theta[0])],
[-np.sin(theta[1]), np.sin(theta[0]) * np.cos(theta[1]), np.cos(theta[0]) * np.cos(theta[1])]
])
t = np.array([pose[0], pose[1], pose[2]])
return np.concatenate((R, t.reshape(-1, 1)), axis=1)
def inverse_kinematics(xyz, xyz_base, urdf_params):
# 假设urdf_params包含d, a, alpha等
n_joints = len(urdf_params['a'])
for j in range(n_joints):
delta_x = xyz[0] - (urdf_params['a'][j] * np.cos(theta_initial[j]) + urdf_params['d'][j])
delta_y = xyz[1] - (urdf_params['a'][j] * np.sin(theta_initial[j]))
if delta_y < 0 or (delta_x**2 + delta_y**2 > (urdf_params['d'][j])**2 and np.abs(np.arctan2(delta_y, delta_x)) > np.pi/2 - urdf_params['alpha'][j]):
raise ValueError("No solution found for the given position")
# 使用线性插值寻找两个相邻关节角之间的解决方案
step_size = 0.01
while True:
theta_intermediate = theta_initial[j] + step_size
H1 = homogeneous_matrix([0, 0, 0], theta_intermediate)
H2 = homogeneous_matrix([urdf_params['d'][j], 0, 0], theta_intermediate)
H = np.linalg.inv(H1.T @ H2)
t = H @ np.array([delta_x, delta_y, 0, 1])
if t[-1] > 0:
# 找到了一个可行的θ值
theta_new = [t[0], t[1], t[2]]
break
else:
step_size *= 2
return theta_new
# 给定末端工具位置(x, y, z) 和 姿态信息
xyz_target = np.array([x, y, z])
xyz_base = np.array([0, 0, 0]) # 基座坐标,此处默认为原点
# 调用逆运动学函数得到关节角度
try:
joint_angles = inverse_kinematics(xyz_target, xyz_base, {'d': d, 'a': a, 'alpha': alpha})
except ValueError as e:
print(e)
print(f"Joint angles: {joint_angles}")
阅读全文
相关推荐
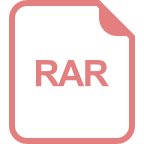
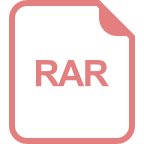

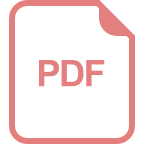
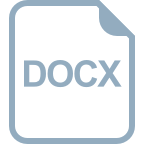
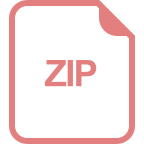
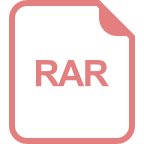
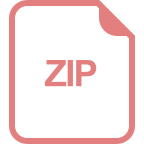
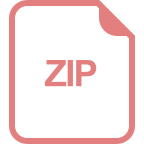
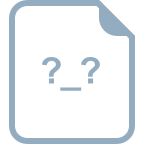
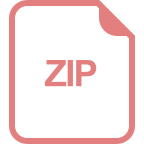
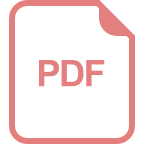
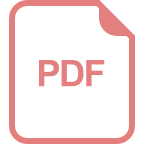
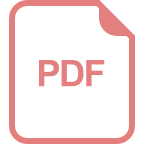