cin>>i; cin>>n; map<int,int> v; for (map<int,int>::iterator it=v.begin();it!=v.end()){ v.insert(v.end(),make_pair(i,n)); },我这么写可以吗
时间: 2024-11-05 21:28:44 浏览: 22
你提供的代码片段是用来插入元素到`map<int, int>`容器`v`中。但是需要注意几个点:
1. `cin >> i;` 和 `cin >> n;` 这两行用于从标准输入读取整数 `i` 和 `n`。如果 `i` 或 `n` 没有正确的值,或者用户输入不符合预期(例如非数字),这可能会导致错误。
2. `map<int,int>::iterator it=v.begin();` 创建了一个迭代器 `it`,它指向映射 `v` 的第一个元素。
3. `while(it!=v.end())` 这里应该是一个循环条件判断,因为你通常不会直接比较迭代器等于结束,而是使用递增操作 (`++it`)。你可能是想在遍历完所有已有的键值对后再插入新的 `(i, n)` 对。
4. `v.insert(v.end(), make_pair(i, n));` 这行代码是在 map 的末尾插入一个新的键值对。如果 `i` 已经存在于 map 中,它会覆盖原来的值;否则,新键值对会被添加。
正确的语法应该是这样的:
```cpp
if (cin >> i >> n) {
// 验证输入有效后才插入
v.insert(v.end(), std::make_pair(i, n));
}
```
同时,考虑到 `cin` 可能失败并返回 false,最好处理异常或者检查输入是否成功。
相关问题
#include <iostream> #include <map> #include <vector> #include <algorithm> void brotherWord(std::vector<std::string> strVect, std::string word, int k) { std::multimap<std::string, int> rtnMap; //存放是兄弟单词的容器 std::vector<std::string>::iterator iter = strVect.begin(); while(iter != strVect.end()) { std::string curWord = *iter; if(curWord != word) //兄弟单词不能一模一样 { std::string tempword = word; std::sort(tempword.begin(), tempword.end()); std::string tempcurword = curWord; std::sort(tempcurword.begin(), tempcurword.end()); if(tempcurword == tempword) //是兄弟单词 { rtnMap.insert(std::pair<std::string, int>(curWord, 1)); } } iter++; } // print(rtnMap); std::vector<std::string> rtnVect; std::multimap<std::string, int>::iterator iterMap = rtnMap.begin(); while(iterMap != rtnMap.end()) { rtnVect.push_back(iterMap->first); iterMap++; } std::cout << rtnVect.size() << std::endl; if(rtnVect.size() > 1) std::cout << rtnVect[k-1] << std::endl; } int main() { int n; std::cin >> n; std::vector<std::string> strVect; while(n--) { std::string temp; std::cin >> temp; strVect.push_back(temp); } std::string word; std::cin >> word; int k; std::cin >> k; brotherWord(strVect, word, k); return 0; }注释
这段代码是一个查找兄弟单词的程序。它首先从输入中读取一个整数n,表示要输入的单词数量。然后依次读取n个单词,存储在一个名为strVect的字符串向量中。接下来,程序读取一个单词word和一个整数k。函数brotherWord将在strVect中查找与word是兄弟单词的单词,并将它们存储在一个名为rtnVect的字符串向量中。如果rtnVect中的单词数量大于1,则输出第k个单词。
整体来说,这段代码实现了一个简单的兄弟单词查找功能。
#include<bits/stdc++.h> using namespace std; int main() { int n,i,j; int a; map<int,int> mp; //set<int> s; cin>>n; for(i=0;i<n;i++){ cin>>a; mp[a]++; //s.insert(a); } map<int,int>::iterator it=mp.begin(); for(;it!=mp.end();it++) { cout<<mp[*it]<<endl; } }这些代码哪里出错了
这段代码存在一些问题:
1. `#include<bits/stdc++.h>` 是非标准的头文件,不建议使用,可以使用 `#include<iostream>` 和 `#include<map>`。
2. 在 `for` 循环中,应该使用 `it->second` 来获取 `map` 中每个键对应的值,而不是 `mp[*it]`。
3. 在输出时,应该输出 `it->second` 而不是 `mp[*it]`。
修改后的代码如下:
```c++
#include <iostream>
#include <map>
using namespace std;
int main() {
int n, a;
map<int, int> mp;
cin >> n;
for (int i = 0; i < n; i++) {
cin >> a;
mp[a]++;
}
map<int, int>::iterator it = mp.begin();
for (; it != mp.end(); it++) {
cout << it->second << endl;
}
return 0;
}
```
希望对你有帮助!
阅读全文
相关推荐
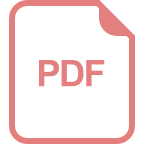
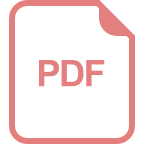
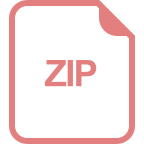






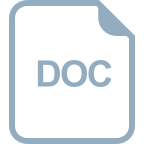
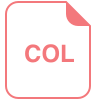
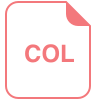




