操作系统实验 哲学家进餐问题C语言代码
时间: 2024-07-16 16:00:42 浏览: 127
操作系统实验中的哲学家进餐问题是一个经典的并发控制问题,用来演示死锁的概念。这个场景中,有五个哲学家围坐在一起,每个人有一根筷子,他们想要交替使用两根筷子吃饭。然而,由于资源分配的顺序问题,如果每个哲学家都试图同时获取左右两根筷子,可能会导致死锁。
在C语言中,实现哲学家进餐问题的代码通常会使用过程(也称为线程)和条件变量来模拟这个场景。以下是一个简单的示例代码:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#define NUM_PHILOSOPHERS 5
#define LEFT_STICK 0
#define RIGHT_STICK 1
struct philosopher {
int id;
pthread_mutex_t sticks[2];
pthread_cond_t conditions;
};
void* philosopher_thread(void* arg) {
struct philosopher* philosopher = (struct philosopher*) arg;
int left, right;
int i;
for (i = 0; i < NUM_PHILOSOPHERS; ++i) {
left = (i + philosopher->id) % NUM_PHILOSOPHERS;
right = (i + philosopher->id + 1) % NUM_PHILOSOPHERS;
printf("Philosopher %d thinking...\n", philosopher->id);
pthread_mutex_lock(&philosopher->sticks[left]);
pthread_cond_wait(&philosopher->conditions[left], &philosopher->sticks[left]);
printf("Philosopher %d got left stick\n", philosopher->id);
pthread_mutex_lock(&philosopher->sticks[right]);
printf("Philosopher %d got right stick and eating\n", philosopher->id);
// Simulate eating for some time
sleep(1);
printf("Philosopher %d finished eating, putting sticks back\n", philosopher->id);
pthread_mutex_unlock(&philosopher->sticks[right]);
pthread_mutex_unlock(&philosopher->sticks[left]);
pthread_cond_signal(&philosopher->conditions[right]);
}
return NULL;
}
int main() {
struct philosopher philosophers[NUM_PHILOSOPHERS];
int i;
for (i = 0; i < NUM_PHILOSOPHERS; ++i) {
pthread_mutex_init(&philosophers[i].sticks[LEFT_STICK], NULL);
pthread_mutex_init(&philosophers[i].sticks[RIGHT_STICK], NULL);
pthread_cond_init(&philosophers[i].conditions[LEFT_STICK], NULL);
pthread_cond_init(&philosophers[i].conditions[RIGHT_STICK], NULL);
}
for (i = 0; i < NUM_PHILOSOPHERS; ++i) {
pthread_create(&philosopher_threads[i], NULL, philosopher_thread, (void*) &philosophers[i]);
}
for (i = 0; i < NUM_PHILOSOPHERS; ++i) {
pthread_join(philosopher_threads[i], NULL);
}
for (i = 0; i < NUM_PHILOSOPHERS; ++i) {
pthread_mutex_destroy(&philosophers[i].sticks[LEFT_STICK]);
pthread_mutex_destroy(&philosophers[i].sticks[RIGHT_STICK]);
pthread_cond_destroy(&philosophers[i].conditions[LEFT_STICK]);
pthread_cond_destroy(&philosophers[i].conditions[RIGHT_STICK]);
}
return 0;
}
阅读全文
相关推荐
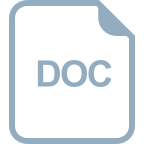
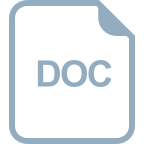
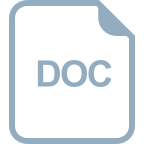
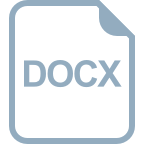
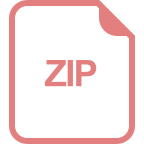
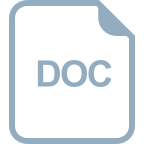
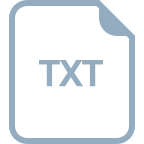
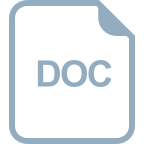
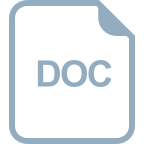

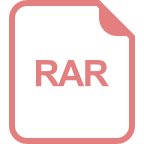
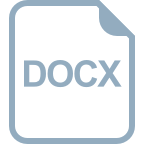
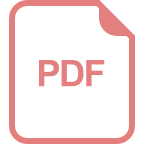
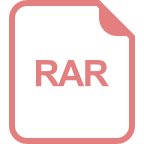
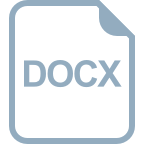
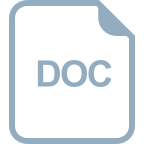
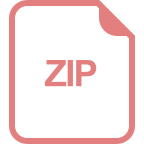
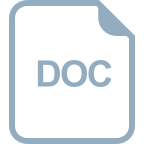
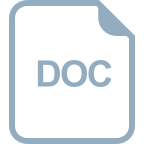