使用eclipse编写一个页面,添加一个商品到数据库,并显示新添加的商品信息
时间: 2023-11-22 22:54:37 浏览: 70
首先,需要确保你已经安装了Eclipse和Java EE插件。接下来,按照以下步骤进行操作:
1. 创建一个动态Web项目。选择File > New > Dynamic Web Project,输入项目名称,选择Target runtime,点击Next。
2. 添加JDBC驱动程序。将JDBC驱动程序添加到Web项目中的lib目录中。
3. 创建一个JSP页面。选择File > New > JSP File,输入文件名称,选择创建位置,点击Finish。
4. 在JSP页面中添加HTML表单元素,包括商品名称、价格、描述等。
5. 创建一个Java Servlet类。选择File > New > Servlet,输入Servlet名称和URL映射,点击Next。
6. 在Servlet类中编写代码,将表单数据插入数据库中,并将新添加的商品信息显示在JSP页面上。
7. 在web.xml文件中配置Servlet类的映射。
8. 运行Web应用程序。
以下是一个简单的示例代码,用于添加商品到数据库并显示新添加的商品信息:
JSP页面(addProduct.jsp):
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Add Product</title>
</head>
<body>
<h1>Add Product</h1>
<form action="AddProductServlet" method="post">
<label for="name">Product Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="price">Price:</label>
<input type="text" id="price" name="price"><br><br>
<label for="description">Description:</label>
<textarea id="description" name="description"></textarea><br><br>
<input type="submit" value="Add Product">
</form>
</body>
</html>
```
Servlet类(AddProductServlet.java):
```java
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/AddProductServlet")
public class AddProductServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
String price = request.getParameter("price");
String description = request.getParameter("description");
Connection conn = null;
PreparedStatement stmt = null;
try {
// 连接数据库
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
// 插入商品信息到数据库
String sql = "INSERT INTO products (name, price, description) VALUES (?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setString(2, price);
stmt.setString(3, description);
stmt.executeUpdate();
// 查询新添加的商品信息
sql = "SELECT * FROM products WHERE name=?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
ResultSet rs = stmt.executeQuery();
rs.next();
// 将商品信息传递到JSP页面并显示
request.setAttribute("product", rs);
request.getRequestDispatcher("product.jsp").forward(request, response);
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
JSP页面(product.jsp):
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Product</title>
</head>
<body>
<h1>Product Information</h1>
<table>
<tr>
<td>Name:</td>
<td><%= request.getAttribute("product").getString("name") %></td>
</tr>
<tr>
<td>Price:</td>
<td><%= request.getAttribute("product").getString("price") %></td>
</tr>
<tr>
<td>Description:</td>
<td><%= request.getAttribute("product").getString("description") %></td>
</tr>
</table>
</body>
</html>
```
注意:上述示例代码仅供参考,实际应用中需要根据具体情况进行修改和调整。同时,需要确保数据库连接信息正确,并且在Web项目的lib目录中导入正确的JDBC驱动程序。
相关推荐
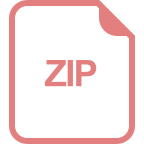
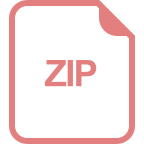
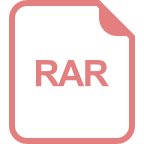















