软件实现建立一个文件夹,在此文件夹中建立一个log记录文件(也是软件实现)。 键盘随机输入一个数,通过子线程把这个数记录到log文件里面去。 同时记录输入数据的时间,并且每次输入的数据不能覆盖前面输入的数据。 编译进程需要添加参数-lpthread
时间: 2023-12-03 11:43:57 浏览: 32
这是一个比较典型的多线程编程问题,需要使用C++的多线程库pthread来实现。下面是一个简单的代码示例:
```cpp
#include <iostream>
#include <fstream>
#include <ctime>
#include <pthread.h>
using namespace std;
const string logFileName = "log.txt";
void* inputThread(void* arg)
{
ofstream logFile(logFileName, ios_base::app);
while (true)
{
int num;
cin >> num;
time_t now = time(0);
tm* localTime = localtime(&now);
logFile << localTime->tm_year + 1900 << "-"
<< localTime->tm_mon + 1 << "-"
<< localTime->tm_mday << " "
<< localTime->tm_hour << ":"
<< localTime->tm_min << ":"
<< localTime->tm_sec << " "
<< num << endl;
}
return NULL;
}
int main()
{
ofstream logFile(logFileName);
pthread_t thread;
int result = pthread_create(&thread, NULL, inputThread, NULL);
if (result != 0)
{
cerr << "Error: Failed to create thread." << endl;
return 1;
}
pthread_join(thread, NULL);
return 0;
}
```
这个程序通过创建一个子线程来处理键盘输入,并将输入的数字和时间戳记录到log文件中。注意,log文件的打开方式是ios_base::app,这会让ofstream在每次写入时都将数据追加到文件末尾,而不是覆盖之前的内容。另外,为了避免多个线程同时访问log文件,我们在主线程中也打开了一次log文件,并在子线程中使用同一个文件流对象进行写入。最后,main函数中调用pthread_join函数等待子线程结束,以避免程序在主线程退出前子线程还在运行的情况。
相关推荐
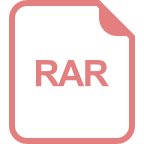
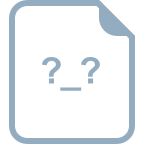
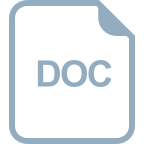














